C#: Create a identity matrix
Create Identity Matrix
In linear algebra, the identity matrix of size n is the n x n square matrix with ones on the main diagonal and zeros elsewhere.
Terminology and notation:
The identity matrix is often denoted by In, or simply by I if the size is immaterial or can be trivially determined by the context.
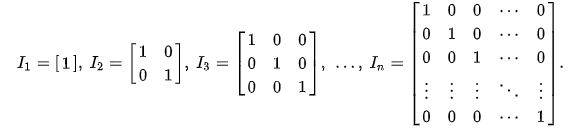
Write a C# Sharp program to create an identity matrix.
Sample Data:
Input a number: 3
1 0 0
0 1 0
0 0 1
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int n;
// Prompt the user to input a number
Console.WriteLine("Input a number:");
// Read the input from the user and convert it to an integer
n = Convert.ToInt32(Console.ReadLine());
// Create a square matrix of size n x n filled with zeroes except for diagonal elements (identity matrix)
var M =
Enumerable.Range(0, n)
.Select(i =>
Enumerable.Repeat(0, n)
.Select((z, j) => j == i ? 1 : 0) // Sets diagonal elements to 1, rest to 0
.ToList()
)
.ToList();
// Display the matrix (identity matrix) generated above
foreach (var row in M)
{
foreach (var element in row)
{
Console.Write(" " + element); // Print each element of the matrix row
}
Console.WriteLine(); // Move to the next line after printing each row
}
}
}
}
Sample Output:
Input a number: 3 1 0 0 0 1 0 0 0 1
Flowchart:
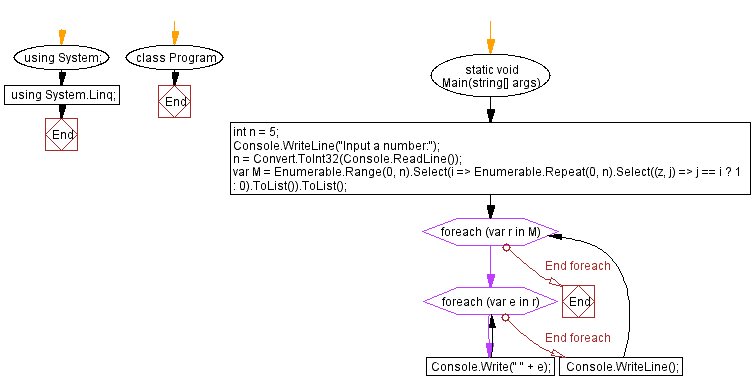
C# Sharp Code Editor:
Previous C# Sharp Exercise: Calculate the value of e (Euler's number).
Next C# Sharp Exercise: Sort characters in a string.
What is the difficulty level of this exercise?