C#: Find the longest word in a string
C# Sharp Basic: Exercise-24 with Solution
Find Longest Word in String
Write a C# program to find the longest word in a string.
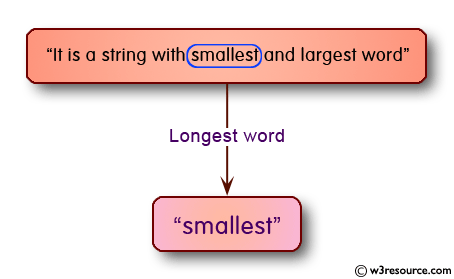
Sample Solution:-
C# Sharp Code:
using System;
// This is the beginning of the Exercise24 class
public class Exercise24
{
// This is the main method where the program execution starts
public static void Main()
{
string line = "Write a C# Sharp Program to display the following pattern using the alphabet.";
// Splitting the string into words based on spaces and storing them in an array
string[] words = line.Split(new[] { " " }, StringSplitOptions.None);
string word = ""; // Initializing an empty string to store the word with the maximum length
int ctr = 0; // Initializing a counter variable to keep track of the maximum length
// Looping through each word in the words array
foreach (String s in words)
{
// Checking if the length of the current word is greater than the stored maximum length
if (s.Length > ctr)
{
word = s; // If the current word's length is greater, update the 'word' variable
ctr = s.Length; // Update the maximum length counter
}
}
Console.WriteLine(word); // Displaying the word with the maximum length
}
}
Test Data: Write a C# Sharp Program to display the following pattern using the alphabet.
Sample Output:
following
Flowchart:
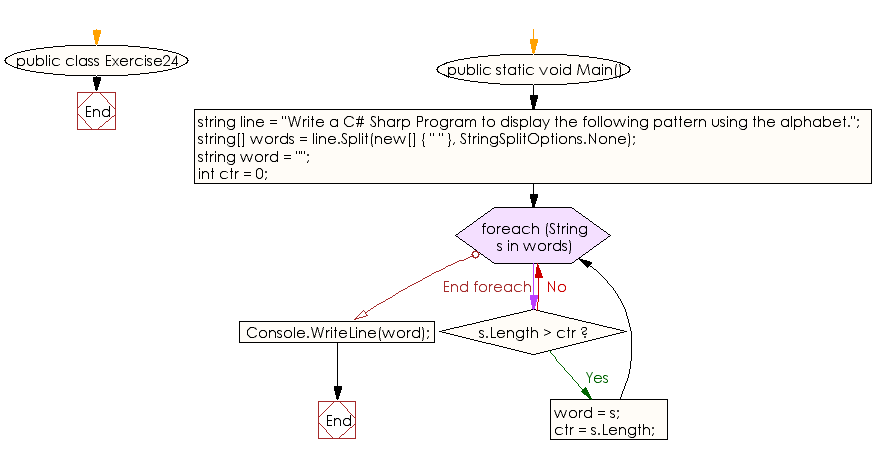
C# Sharp Code Editor:
Previous: Write a C# program to convert a given string into lowercase.
Next: Write a C# program to print the odd numbers from 1 to 99. Prints one number per line.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics