C#: Reverse the words of a sentence
Reverse Words in Sentence
Write a C# program to reverse the words of a sentence.
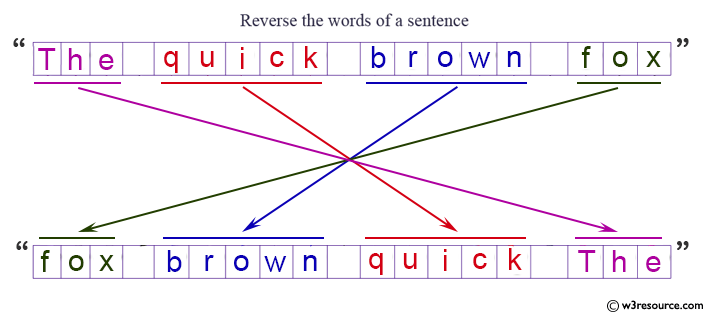
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
// This is the beginning of the Exercise28 class
public class Exercise28 {
// This is the main method where the program execution starts
public static void Main() {
string line = "Display the pattern like pyramid using the alphabet.";
Console.WriteLine("\nOriginal String: " + line); // Displaying the original string
string result = ""; // Initializing an empty string to store the reversed words
List<string> wordsList = new List<string>(); // Creating a list to store reversed strings
string[] words = line.Split(new[] { " " }, StringSplitOptions.None); // Splitting the string into individual words
// Loop to reverse the words and create a new string
for (int i = words.Length - 1; i >= 0; i--) {
result += words[i] + " "; // Building the reversed string by adding words in reverse order
}
wordsList.Add(result); // Adding the reversed string to the list
// Loop to print the reversed string from the list
foreach (String s in wordsList) {
Console.WriteLine("\nReverse String: " + s); // Displaying the reversed string
}
}
}
Sample Output:
Original String: Display the pattern like pyramid using the alphabet. Reverse String: alphabet. the using pyramid like pattern the Display
Flowchart:
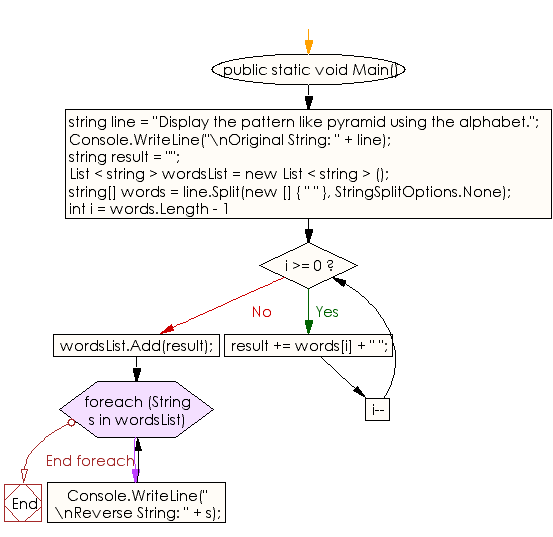
C# Sharp Code Editor:
Previous: Write a C# program and compute the sum of the digits of an integer.
Next: Write a C# program to find the size of a specified file in bytes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.