C#: Check if a string starts with a specified word
Check String Starts with Word
Write a C# program to check if a string starts with a specified word.
Note: Suppose the sentence starts with "Hello"
Sample Data: string1 = "Hello how are you?"
Result: Hello.
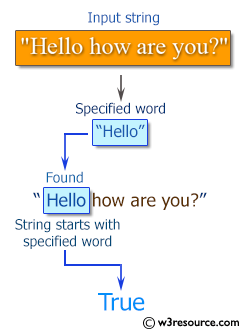
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise34 {
static void Main(string[] args) {
// Declare a string variable 'str' to store user input
string str;
// Display a message asking the user to input a string
Console.Write("Input a string : ");
// Read user input and store it in the 'str' variable
str = Console.ReadLine();
// Check conditions and output result based on string content
// Check if the length of the string is less than 6 and it's equal to "Hello"
// OR check if the string starts with "Hello" and the character at index 5 is a space (' ')
Console.WriteLine((str.Length < 6 && str.Equals("Hello")) || (str.StartsWith("Hello") && str[5] == ' '));
}
}
Sample Output:
Input a string : Hello how are you? True
Flowchart:
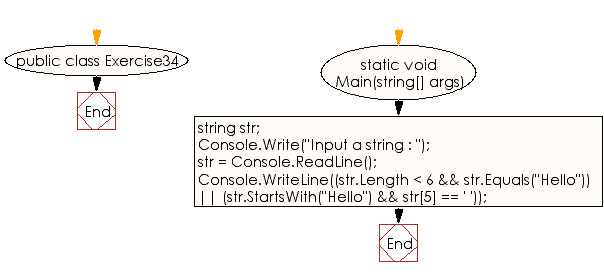
C# Sharp Code Editor:
Previous: Write a C# program to check if a given positive number is a multiple of 3 or a multiple of 7.
Next: Write a C# program to check two given numbers where one is less than 100 and other is greater than 200.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.