C#: Check if "HP" appears at second position in a string and returns the string without "HP"
Remove 'HP' from String
Write a C# program to check if "HP" appears at the second position in a string and return the string without "HP".
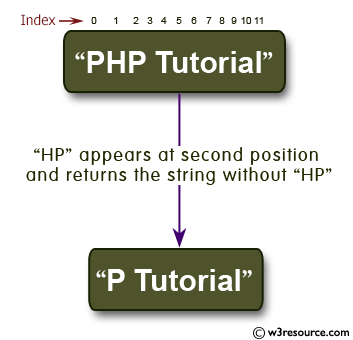
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise37 {
static void Main(string[] args) {
// Define a string variable 'str' and assign it the value "PHP Tutorial"
string str = "PHP Tutorial";
// Check if the substring from index 1 of length 2 in 'str' equals "HP"
// If true, remove the substring "HP" starting from index 1; otherwise, keep the original string 'str'
Console.WriteLine((str.Substring(1, 2).Equals("HP") ? str.Remove(1, 2) : str));
}
}
Sample Output:
P Tutorial
Flowchart:
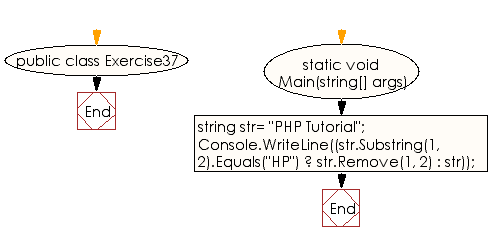
C# Sharp Code Editor:
Previous: Write a C# program to check if an integer (from the two given integers) is in the range -10 to 10.
Next: Write a C# program to get a new string of two characters from a given string. The first and second character of the given string must be "P" and "H", so PHP will be "PH".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.