C#: Print the result of print the result of the specified operations
Specified Operations Results
Write a C# Sharp program to print the results of the specified operations.
C# Sharp: Working with Arithmetic Expression
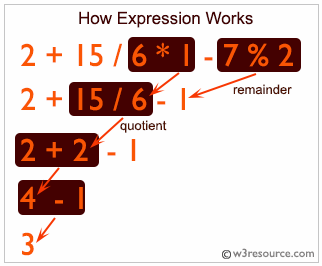
Sample Solution:
C# Sharp Code:
// This is the beginning of the Exercise4 class
public class Exercise4
{
// This is the main method where the program execution starts
public static void Main()
{
// This line performs arithmetic operations (-1 + 4 * 6) and outputs the result to the console
System.Console.WriteLine(-1 + 4 * 6); // -1 + 24 = 23
// This line performs arithmetic operations ((35 + 5) % 7) and outputs the result to the console
System.Console.WriteLine((35 + 5) % 7); // 40 % 7 = 5 (remainder of 40 divided by 7)
// This line performs arithmetic operations (14 + (-4 * 6) / 11) and outputs the result to the console
System.Console.WriteLine(14 + -4 * 6 / 11); // 14 - (24 / 11) = 14 - 2 = 12
// This line performs arithmetic operations (2 + 15 / 6 * 1 - 7 % 2) and outputs the result to the console
System.Console.WriteLine(2 + 15 / 6 * 1 - 7 % 2); // 2 + (15 / 6) - (7 % 2) = 2 + 2 - 1 = 4 - 1 = 3
}
}
Sample Output:
23 5 12 3
Flowchart:
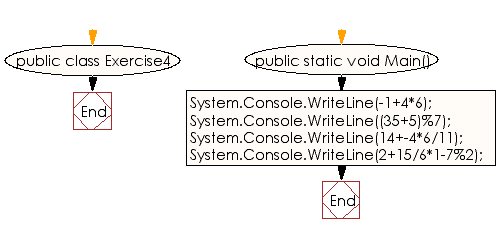
C# Sharp Code Editor:
Previous: Write a C# Sharp program to print the result of dividing two numbers.
Next: Write a C# Sharp program to swap two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.