C#: Check if the first element and the last element are equal of an array of integers
First and Last Element Equal in Array
Write a C# program that checks if the first element and the last element of an array of integers are equal. The array length is 1 or more.
Pictorial Presentation:
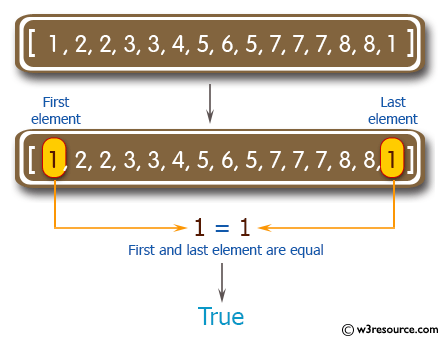
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise48
{
public static void Main()
{
// Define an array of integers 'nums' with pre-defined values
int[] nums = {1, 2, 2, 3, 3, 4, 5, 6, 5, 7, 7, 7, 8, 8, 1};
// Check if the length of the 'nums' array is greater than or equal to 1
// AND if the first element of the array is equal to the last element of the array
// Print the result of the logical AND operation between these conditions
Console.WriteLine((nums.Length >= 1) && (nums[0].Equals(nums[nums.Length - 1])));
}
}
Sample Output:
Array1: [1, 2, 2, 3, 3, 4, 5, 6, 5, 7, 7, 7, 8, 8, 1] True
Flowchart:
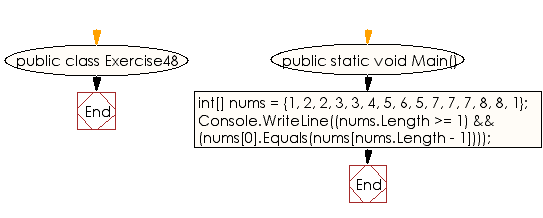
C# Sharp Code Editor:
Previous: Write a C# program to compute sum of all the elements of an array of integers.
Next: Write a C# program to check if the first element or the last element of the two arrays ( length 1 or more) are equal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.