C#: Rotate an array of integers in left direction
C# Sharp Basic: Exercise-50 with Solution
Rotate Array Left
Write a C# program to rotate an array (length 3) of integers in the left direction.
Pictorial Presentation:
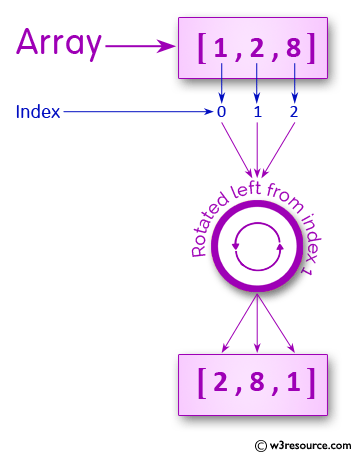
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Exercise50
{
public static void Main()
{
// Define an array of integers 'nums' with pre-defined values
int[] nums = {1, 2, 8};
// Display the elements of 'nums' array using string.Join to concatenate them
Console.WriteLine("\nArray1: [{0}]", string.Join(", ", nums));
var temp = nums[0]; // Store the first element of 'nums' in a temporary variable 'temp'
// Iterate through the elements of the 'nums' array (except the last element) using a for loop
for (var i = 0; i < nums.Length - 1; i++)
{
nums[i] = nums[i + 1]; // Shift each element one place to the left
}
nums[nums.Length - 1] = temp; // Assign the value of 'temp' to the last element of 'nums'
// Display the elements of the modified 'nums' array after rotation
Console.WriteLine("\nAfter rotating array becomes: [{0}]", string.Join(", ", nums));
}
}
Sample Output:
Array1: [1, 2, 8] After rotating array becomes: [2, 8, 1]
Flowchart:
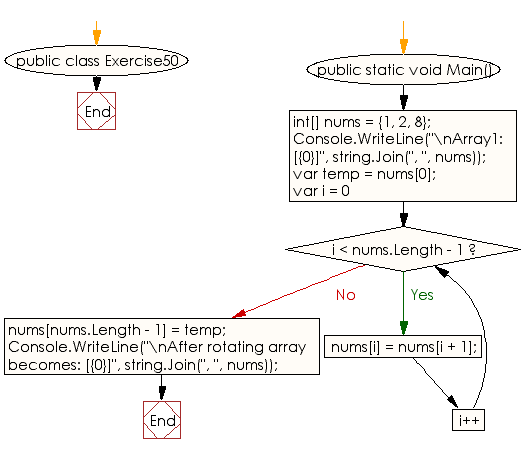
C# Sharp Code Editor:
Previous: Write a C# program to check if the first element or the last element of the two arrays ( length 1 or more) are equal.
Next: Write a C# program to get the larger value between first and last element of an array (length 3) of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-50.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics