C#: Print the output of multiplication of three numbers which will be entered by the user
Multiply Three Numbers
Write a C# Sharp program to print the output of the multiplication of three numbers entered by the user.
C# Sharp multiplication (*) operator :
The multiplication operator (*), which computes the product of its operands. Also, the dereference operator, which allows reading and writing to a pointer.
Note:
- All numeric types have predefined multiplication operators.
- The * operator is also used to declare pointer types and to dereference pointers.
- User-defined types can overload the binary * operator. When a binary operator is overloaded, the corresponding assignment operator, if any, is also implicitly overloaded.
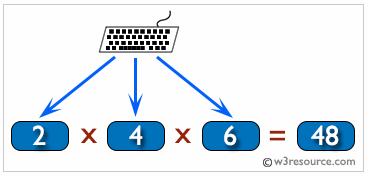
Sample Solution:
C# Sharp Code:
using System;
// This is the beginning of the Exercise6 class
public class Exercise6
{
// This is the main method where the program execution starts
public static void Main()
{
// Declaration of variables to store three numbers
int num1, num2, num3;
// Prompting the user to input the first number to multiply
Console.Write("Input the first number to multiply: ");
// Reading the first number entered by the user and converting it to an integer
num1 = Convert.ToInt32(Console.ReadLine());
// Prompting the user to input the second number to multiply
Console.Write("Input the second number to multiply: ");
// Reading the second number entered by the user and converting it to an integer
num2 = Convert.ToInt32(Console.ReadLine());
// Prompting the user to input the third number to multiply
Console.Write("Input the third number to multiply: ");
// Reading the third number entered by the user and converting it to an integer
num3 = Convert.ToInt32(Console.ReadLine());
// Calculating the result of multiplying the three numbers
int result = num1 * num2 * num3;
// Displaying the multiplication formula and the result to the console
Console.WriteLine("Output: {0} x {1} x {2} = {3}",
num1, num2, num3, result);
}
}
Sample Output:
Input the first number to multiply: 2 Input the second number to multiply: 8 Input the third number to multiply: 5 Output: 2 x 8 x 5 = 80
Flowchart:
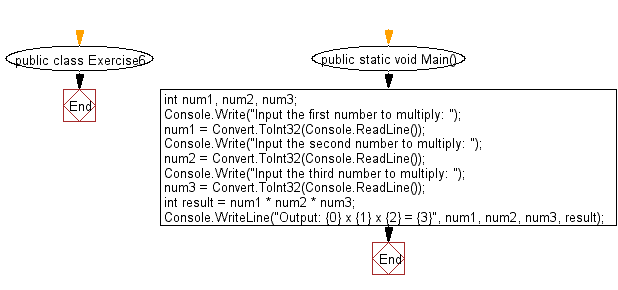
C# Sharp Code Editor:
Previous: Write a C# Sharp program to swap two numbers.
Next: Write a C# Sharp program to print on screen the output of adding, subtracting, multiplying and dividing of two numbers which will be entered by the user.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.