C#: Calculate the sum of all the integers of a rectangular matrix except those integers which are located below an intger of value 0
Sum of Matrix with Zero Condition
Write a C# program to calculate the sum of all integers in a rectangular matrix. However, exclude those integers located below an integer of value 0.
Sample Example:
matrix = [[0, 2, 3, 2],
[0, 6, 0, 1],
[4, 0, 3, 0]]
Eligible integers which will be participated to calculate the sum -
matrix = [[X, 2, 3, 2],
[X, 6, X, 1],
[X, X, X, X]]
Therefore sum will be: 2 + 3 + 2 + 6 + 1 = 14
Sample Solution:
C# Sharp Code:
using System;
public class Example
{
// Function to calculate the sum of matrix elements meeting certain conditions
public static int sum_matrix_elements(int[][] my_matrix)
{
int x = 0; // Initialize a variable to store the sum of selected matrix elements
// Loop through each column of the matrix
for (int i = 0; i < my_matrix[0].Length; i++)
{
// Iterate through each row in the current column until a non-positive element is encountered
for (int j = 0; j < my_matrix.Length && my_matrix[j][i] > 0; j++)
{
// Add the positive matrix element to the sum
x += my_matrix[j][i];
}
}
// Return the sum of matrix elements meeting the condition (positive elements)
return x;
}
// Main method to test the sum_matrix_elements function
public static void Main()
{
// Testing the sum_matrix_elements function with different input matrices
Console.WriteLine(sum_matrix_elements(
new int[][] {
new int[]{0, 2, 3, 2},
new int[]{0, 6, 0, 1},
new int[]{4, 0, 3, 0}
})); // Output: 17
Console.WriteLine(sum_matrix_elements(
new int[][] {
new int[]{1, 2, 1, 0 },
new int[]{0, 5, 0, 0},
new int[]{1, 1, 3, 10 }
})); // Output: 24
Console.WriteLine(sum_matrix_elements(
new int[][] {
new int[]{1, 1},
new int[]{2, 2},
new int[]{3, 3},
new int[]{4, 4}
})); // Output: 10
}
}
Sample Output:
14 10 20
Flowchart:
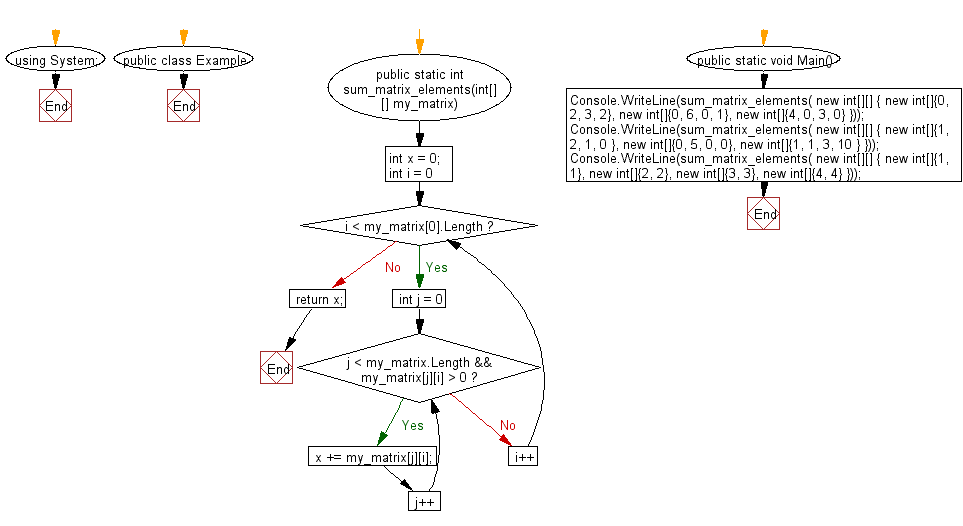
For more Practice: Solve these Related Problems:
- Write a C# program to calculate the sum of a matrix excluding numbers below a negative value instead of zero.
- Write a C# program to compute the total sum of a matrix excluding all elements in rows containing at least one zero.
- Write a C# program to replace all elements below zero values with -1 before summing the matrix.
- Write a C# program to sum all matrix values that are not adjacent (horizontally or vertically) to any zero.
Go to:
PREV : Check Strictly Increasing Sequence.
NEXT : Sort Integers Keeping -5 Fixed.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.