C#: Verify that a string contains valid parentheses
Validate Brackets in String
A given string contains the bracket characters '(', ')', '{', '}', '<', ‘>', '[' and ']',
Write a C# programme to check the said string is valid or not. The input string will be valid when open brackets and closed brackets are same type of brackets.
Or
open brackets will be closed in proper order.
Sample Data:
( "<>") -> True
("<>()[]{}") -> True
("(<>") -> False
("[<>()[]{}]") -> True
Sample Solution-1:
C# Sharp Code:
using System;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing different strings containing various parentheses combinations
string text = "<>";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "<>()[]{}";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "(<>";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "[<>()[]{}]";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
}
// Method to verify if a string contains valid parentheses
public static bool test(string s)
{
// Creating a stack to store opening parentheses
Stack<char> ch = new Stack<char>();
// Iterating through each character in the input string
foreach (var item in s.ToCharArray())
{
// Checking the type of parentheses and pushing the respective closing parentheses onto the stack
if (item == '(')
ch.Push(')');
else if (item == '<')
ch.Push('>');
else if (item == '[')
ch.Push(']');
else if (item == '{')
ch.Push('}');
else if (ch.Count == 0 || ch.Pop() != item)
return false; // If the closing parentheses don't match the top of the stack or stack is empty, return false
}
// If the stack is empty after processing all characters, return true, otherwise return false
return ch.Count == 0;
}
}
}
Sample Output:
Original string: <> Verify the said string contains valid parentheses: True Original string: <>()[]{} Verify the said string contains valid parentheses: True Original string: (<> Verify the said string contains valid parentheses: False Original string: [<>()[]{}] Verify the said string contains valid parentheses: True
Flowchart:
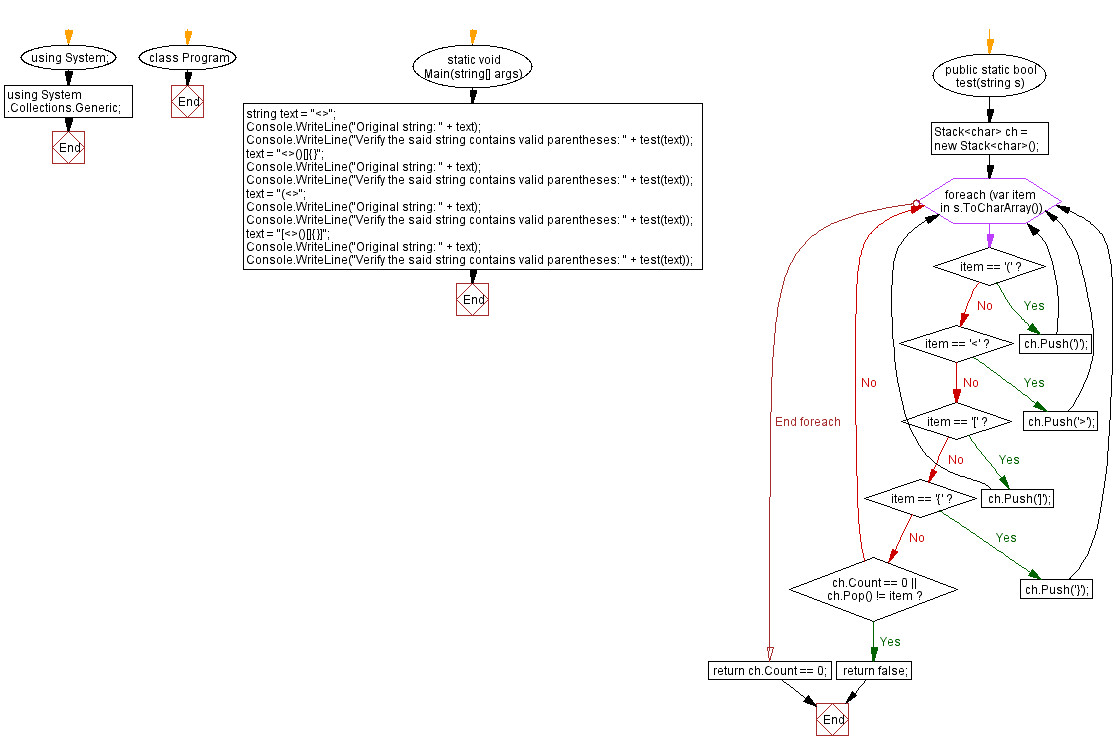
Sample Solution-2:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing different strings containing various parentheses combinations
string text = "<>";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "<>()[]{}";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "(<>";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
text = "[<>()[]{}]";
Console.WriteLine("Original string: " + text);
Console.WriteLine("Verify the said string contains valid parentheses: " + test(text));
}
// Method to verify if a string contains valid parentheses
public static bool test(string text)
{
string temp_text = string.Empty;
// Loop continues until the text doesn't change anymore after replacements
while (text != temp_text)
{
temp_text = text;
// Replacing pairs of parentheses with an empty string in each iteration
text = text.Replace("<>", "").Replace("()", "").Replace("[]", "").Replace("{}", "");
}
// If the final text is empty, all parentheses are balanced, return true; otherwise, return false
return text == string.Empty ? true : false;
}
}
}
Sample Output:
Original string: <> Verify the said string contains valid parentheses: True Original string: <>()[]{} Verify the said string contains valid parentheses: True Original string: (<> Verify the said string contains valid parentheses: False Original string: [<>()[]{}] Verify the said string contains valid parentheses: True
Flowchart:
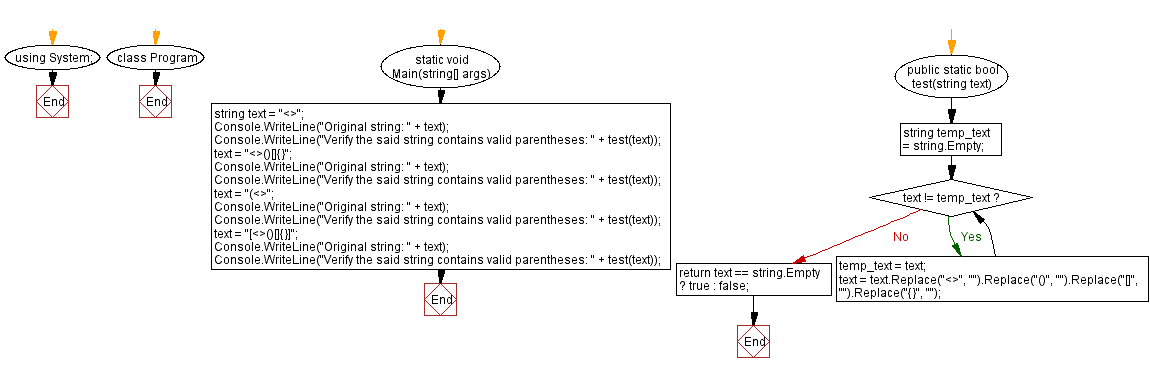
C# Sharp Code Editor:
Previous C# Sharp Exercise: Find the longest common prefix from an array of strings.
Next C# Sharp Exercise: String with same characters .
What is the difficulty level of this exercise?