C#: Calculate root of Quadratic Equation
Write a C# Sharp program to calculate the root of a quadratic equation.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise11 // Declaration of the Exercise11 class
{
public static void Main() // Entry point of the program
{
int a, b, c; // Declaration of integer variables a, b, and c for quadratic equation coefficients
double d, x1, x2; // Declaration of double variables d, x1, and x2 for discriminant and roots
Console.Write("\n\n"); // Printing new lines
Console.Write("Calculate root of Quadratic Equation :\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the value of a : "); // Prompting user to input the value of coefficient a
a = Convert.ToInt32(Console.ReadLine()); // Reading the input value of coefficient a from the user
Console.Write("Input the value of b : "); // Prompting user to input the value of coefficient b
b = Convert.ToInt32(Console.ReadLine()); // Reading the input value of coefficient b from the user
Console.Write("Input the value of c : "); // Prompting user to input the value of coefficient c
c = Convert.ToInt32(Console.ReadLine()); // Reading the input value of coefficient c from the user
d = b * b - 4 * a * c; // Calculating the discriminant
if (d == 0) // Checking if the discriminant is equal to 0
{
Console.Write("Both roots are equal.\n"); // Printing a message if both roots are equal
x1 = -b / (2.0 * a); // Calculating the root when discriminant is zero
x2 = x1; // Assigning the same root to x2
Console.Write("First Root Root1= {0}\n", x1); // Printing the root when discriminant is zero
Console.Write("Second Root Root2= {0}\n", x2); // Printing the root when discriminant is zero
}
else if (d > 0) // Checking if the discriminant is greater than 0
{
Console.Write("Both roots are real and different.\n"); // Printing a message if roots are real and different
x1 = (-b + Math.Sqrt(d)) / (2 * a); // Calculating the first root
x2 = (-b - Math.Sqrt(d)) / (2 * a); // Calculating the second root
Console.Write("First Root Root1= {0}\n", x1); // Printing the first root
Console.Write("Second Root Root2= {0}\n", x2); // Printing the second root
}
else
{
Console.Write("Roots are imaginary;\nNo Solution. \n\n"); // Printing a message if roots are imaginary
}
}
}
Sample Output:
Calculate root of Quadratic Equation : ---------------------------------------- Input the value of a : 1 Input the value of b : 2 Input the value of c : 1 Both roots are equal. First Root Root1= -1 Second Root Root2= -1
Visual Presentation:
Flowchart:
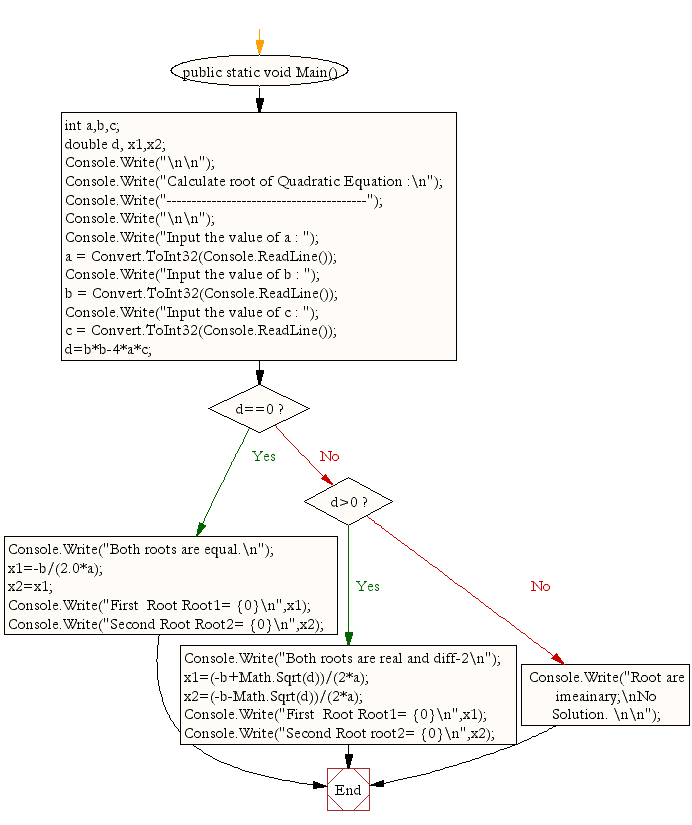
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find the eligibility of admission for a professional course based on the specific criteria.
Next: Write a C# Sharp program to read roll no, name and marks of three subjects and calculate the total, percentage and division.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.