C#: Accept a temperature in centigrade and display a suitable message
C# Sharp Conditional Statement : Exercise-13 with Solution
Write a C# Sharp program to read temperature in centigrade and display a suitable message according to the temperature state below:
Temp < 0 then Freezing weatherTemp 0-10 then Very Cold weather
Temp 10-20 then Cold weather
Temp 20-30 then Normal in Temp
Temp 30-40 then Its Hot
Temp >=40 then Its Very Hot
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise13 // Declaration of the Exercise13 class
{
public static void Main() // Entry point of the program
{
int tmp; // Declaration of an integer variable to store temperature
Console.Write("\n\n"); // Printing new lines
Console.Write("Accept a temperature in centigrade and display a suitable message:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input days temperature : "); // Prompting user to input the temperature
tmp = Convert.ToInt32(Console.ReadLine()); // Reading the input temperature from the user
// Checking the temperature range and displaying suitable messages based on different temperature conditions
if (tmp < 0)
Console.Write("Freezing weather.\n"); // Printing a message for freezing weather
else if (tmp < 10)
Console.Write("Very cold weather.\n"); // Printing a message for very cold weather
else if (tmp < 20)
Console.Write("Cold weather.\n"); // Printing a message for cold weather
else if (tmp < 30)
Console.Write("Normal in temp.\n"); // Printing a message for normal temperature
else if (tmp < 40)
Console.Write("It's Hot.\n"); // Printing a message for hot weather
else
Console.Write("It's very hot.\n"); // Printing a message for very hot weather
}
}
Sample Output:
Accept a temperature in centigrade and display a suitable message: -------------------------------------------------------------------- Input days temperature : 35 Its Hot.
Visual Presentation:
Flowchart:
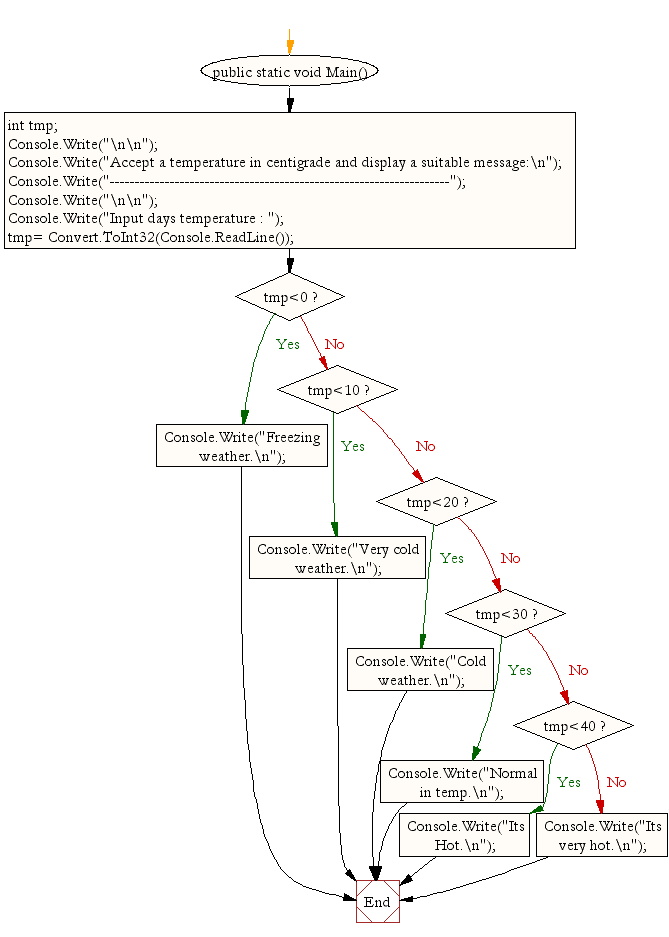
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to read roll no, name and marks of three subjects and calculate the total, percentage and division.
Next: Write a C# Sharp program to check whether a triangle is Equilateral, Isosceles or Scalene.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/conditional-statement/csharp-conditional-statement-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics