C# Sharp Exercises: Calculate Electricity Bill
Write a C# Sharp program to calculate and print the electricity bill of a customer. From the keyboard, the customer's name, ID, and unit consumed should be taken and displayed along with the total amount due.
The charge are as follow:
Unit | Charge/unit |
---|---|
upto 199 | @1.20 |
200 and above but less than 400 | @1.50 |
400 and above but less than 600 | @1.80 |
600 and above | @2.00 |
If bill exceeds Rs. 400 then a surcharge of 15% will be charged and the minimum bill should be of Rs. 100/-
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class exercise18 // Declaration of the exercise18 class
{
static void Main(string[] args) // Entry point of the program
{
int custid, conu; // Declaration of variables to store customer ID and consumed units
double chg, surchg = 0, gramt, netamt; // Declaration of variables for charges, surcharge, gross amount, and net amount
string connm; // Declaration of variable to store customer name
Console.Write("\n\n"); // Printing new lines
Console.Write("Calculate Electricity Bill:\n"); // Displaying the purpose of the program
Console.Write("----------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Customer ID :"); // Prompting user to input customer ID
custid = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
Console.Write("Input the name of the customer :"); // Prompting user to input customer name
connm = Console.ReadLine(); // Reading the input for customer name
Console.Write("Input the unit consumed by the customer : "); // Prompting user to input consumed units
conu = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
// Determining the charge based on consumed units
if (conu < 200)
chg = 1.20;
else if (conu >= 200 && conu < 400)
chg = 1.50;
else if (conu >= 400 && conu < 600)
chg = 1.80;
else
chg = 2.00;
gramt = conu * chg; // Calculating gross amount
if (gramt > 300)
surchg = gramt * 15 / 100.0; // Calculating surcharge if the gross amount exceeds 300
netamt = gramt + surchg; // Calculating net amount
if (netamt < 100)
netamt = 100; // Setting minimum net amount as 100
// Printing electricity bill details
Console.Write("\nElectricity Bill\n");
Console.Write("Customer IDNO :{0}\n", custid);
Console.Write("Customer Name :{0}\n", connm);
Console.Write("unit Consumed :{0}\n", conu);
Console.Write("Amount Charges @Rs. {0} per unit :{1}\n", chg, gramt);
Console.Write("Surchage Amount :{0}\n", surchg);
Console.Write("Net Amount Paid By the Customer :{0}\n", netamt);
}
}
Sample Output:
Calculate Electricity Bill: ---------------------------- Input Customer ID :10001 Input the name of the customer :james Input the unit consumed by the customer : 800 Electricity Bill Customer IDNO :10001 Customer Name :james unit Consumed :800 Amount Charges @Rs. 2 per unit :1600 Surchage Amount :240 Net Amount Paid By the Customer :1840
Flowchart:
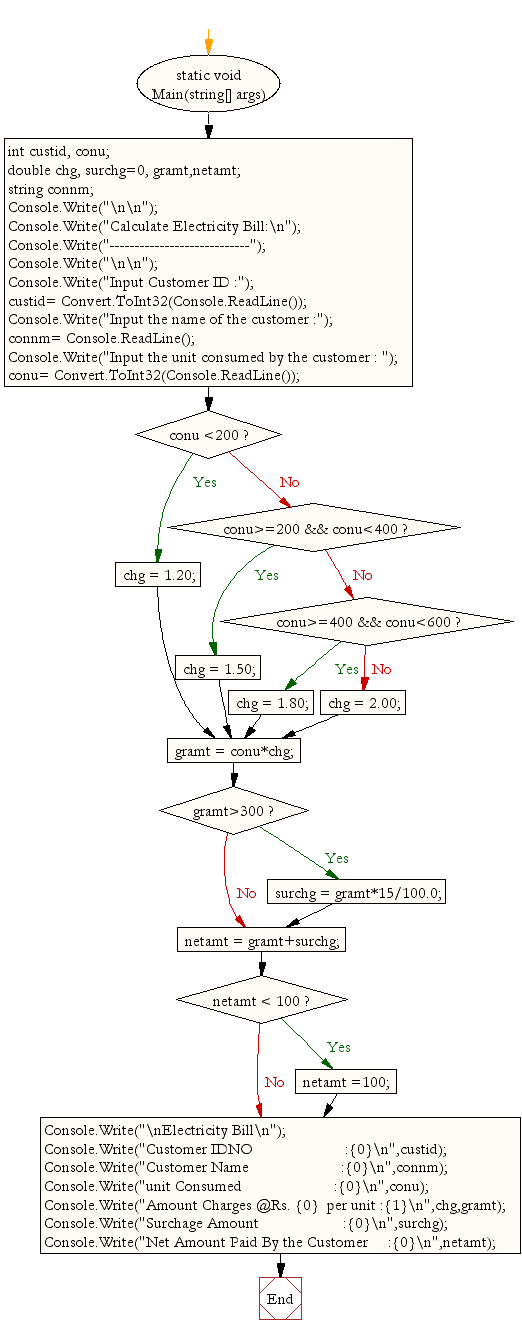
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to calculate profit and loss on a transaction.
Next: Write a program in C# Sharp to accept a grade and display the equivalent description.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.