C#: Accept digit and display in the word
Write a program in C# Sharp to read any digit, display in the word.
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespaces
public class Exercise21 // Declaration of the Exercise21 class
{
public static void Main() // Main method, entry point of the program
{
int cdigit; // Declaration of a variable to store the digit
Console.Write("\n\n"); // Displaying new lines
Console.Write("Accept digit and display in word:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Digit(0-9) : "); // Prompting user to input a digit
cdigit = Convert.ToInt32(Console.ReadLine()); // Reading the input and converting it to an integer
switch(cdigit) // Switch statement based on the entered digit
{
case 0:
Console.Write("Zero\n"); // Displaying "Zero" for digit 0
break;
case 1:
Console.Write("One\n"); // Displaying "One" for digit 1
break;
case 2:
Console.Write("Two\n"); // Displaying "Two" for digit 2
break;
case 3:
Console.Write("Three\n"); // Displaying "Three" for digit 3
break;
case 4:
Console.Write("Four\n"); // Displaying "Four" for digit 4
break;
case 5:
Console.Write("Five\n"); // Displaying "Five" for digit 5
break;
case 6:
Console.Write("Six\n"); // Displaying "Six" for digit 6
break;
case 7:
Console.Write("Seven\n"); // Displaying "Seven" for digit 7
break;
case 8:
Console.Write("Eight\n"); // Displaying "Eight" for digit 8
break;
case 9:
Console.Write("Nine\n"); // Displaying "Nine" for digit 9
break;
default:
Console.Write("Invalid digit. \nPlease try again ....\n"); // Handling invalid digits
break;
}
}
}
Sample Output:
Accept digit and display in word: ----------------------------------- Input Digit(0-9) : 8 Eight
Visual Presentation:
Flowchart:
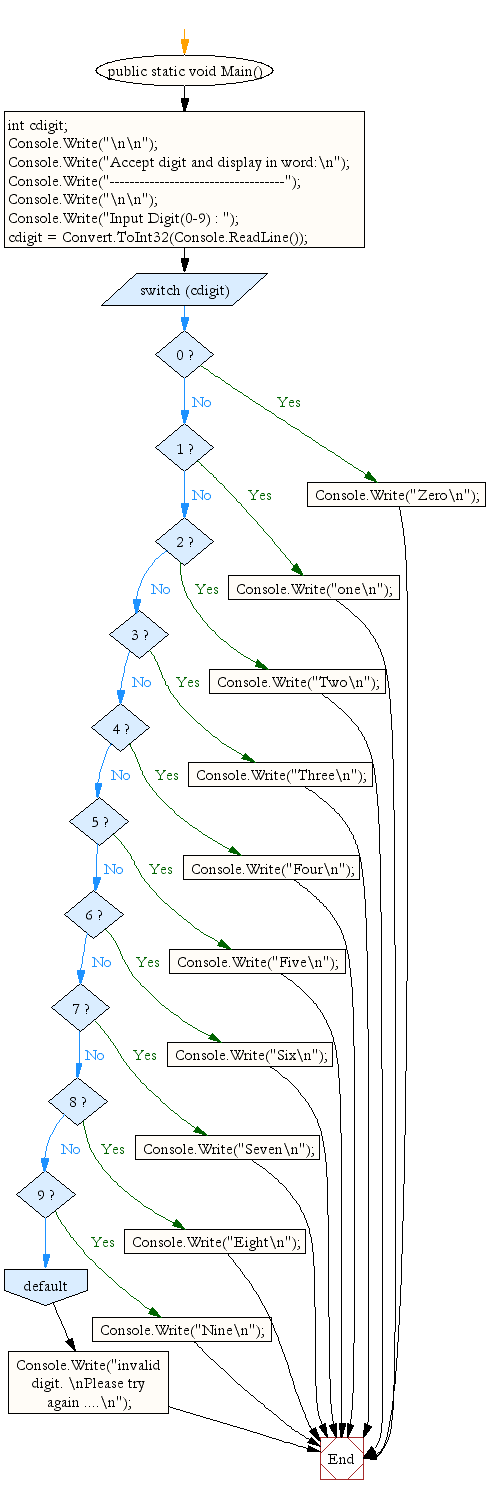
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read any day number in integer and display day name in the word.
Next: Write a program in C# Sharp to read any Month Number in integer and display Month name in the word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.