C#: Determine a specific age is eligible for casting the vote
Write a C# Sharp program to read age of a candidate and determine whether it is eligible for casting his/her own vote.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise5 // Declaration of the Exercise5 class
{
public static void Main() // Entry point of the program
{
int vote_age; // Declaration of the integer variable vote_age
Console.Write("\n\n"); // Printing new lines
Console.Write("Detrermine a specific age is eligible for casting the vote:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Printing new lines
Console.Write("Input the age of the candidate : "); // Prompting user to input the age
vote_age = Convert.ToInt32(Console.ReadLine()); // Reading the input age from the user
if (vote_age < 18) // Checking if the age is less than 18
{
Console.Write("Sorry, You are not eligible to caste your vote.\n"); // Printing a message if not eligible
Console.Write("You would be able to caste your vote after {0} year.\n\n", 18 - vote_age); // Calculating the years remaining to be eligible
}
else
{
Console.Write("Congratulation! You are eligible for casting your vote.\n\n"); // Printing a message if eligible
}
}
}
Sample Output:
Detrermine a specific age is eligible for casting the vote: ---------------------------------------------------------- Input the age of the candidate : 18 Congratulation! You are eligible for casting your vote.
Visual Presentation:
Flowchart:
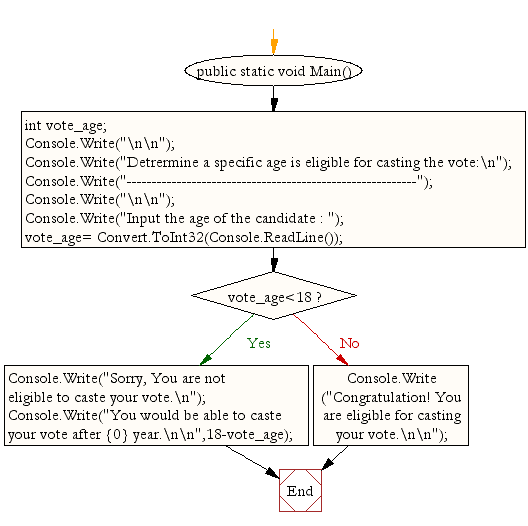
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find whether a given year is a leap year or not.
Next: Write a C# Sharp program to read the value of an integer m and display the value of n is 1 when m is larger than 0, 0 when m is 0 and -1 when m is less than 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.