C#: Calculate the speed in kilometres and miles per hour from distance and time
Write a C# Sharp program that takes distance and time as input and displays speed in kilometres per hour and miles per hour.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise7
{
public static void Main()
{
// Declare variables to store distance, time in hours, minutes, and seconds
float distance;
float hour, min, sec;
// Declare variables to store time in seconds, speed in meters/sec, km/h, and miles/h
float timeSec;
float mps;
float kph, mph;
// Input distance in meters
Console.Write("Input distance (metres): ");
distance = Convert.ToSingle(Console.ReadLine());
// Input time in hours, minutes, and seconds
Console.Write("Input time (hour): ");
hour = Convert.ToSingle(Console.ReadLine());
Console.Write("Input time (minutes): ");
min = Convert.ToSingle(Console.ReadLine());
Console.Write("Input time (seconds): ");
sec = Convert.ToSingle(Console.ReadLine());
// Calculate total time in seconds
timeSec = (hour * 3600) + (min * 60) + sec;
// Calculate speed in meters per second, kilometers per hour, and miles per hour
mps = distance / timeSec;
kph = (distance / 1000.0f) / (timeSec / 3600.0f);
mph = kph / 1.609f;
// Display the calculated speeds
Console.WriteLine("Your speed in meters/sec is {0}", mps);
Console.WriteLine("Your speed in km/h is {0}", kph);
Console.WriteLine("Your speed in miles/h is {0}", mph);
}
}
Sample Output:
Input distance(metres): 10000 Input timeSec(hour): 1 Input timeSec(minutes): 35 Input timeSec(seconds): 5 Your speed in metres/sec is 1.752848 Your speed in km/h is 6.310254 Your speed in miles/h is 3.921849
Visual Presentation:
Flowchart:
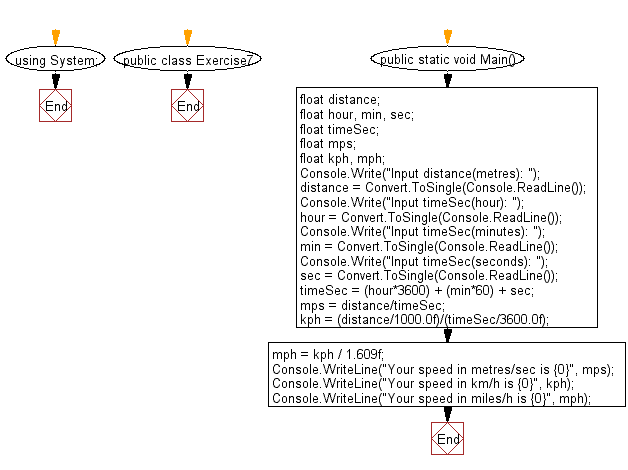
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to display certain values of the function x = y2 + 2y + 1 (using integer numbers for y , ranging from -5 to +5).
Next: Write a C# Sharp program that takes the radius of a sphere as input and calculate and display the surface and volume of the sphere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.