C#: Compare the current date with a given date
Write a C# Sharp program to compare the current date with a given date.
Sample Solution:-
C# Sharp Code:
using System;
public class Example18
{
// Main method, entry point of the program
public static void Main()
{
// Create a DateTime instance for the specific date, 28th July of the current year
System.DateTime theDay = new System.DateTime(System.DateTime.Today.Year, 7, 28);
int compareValue; // Declare a variable to hold comparison result
try
{
// Compare the specific date to today's date
compareValue = theDay.CompareTo(DateTime.Today);
}
catch (ArgumentException)
{
// Catch an ArgumentException in case the value is not a DateTime
Console.WriteLine("Value is not a DateTime");
return;
}
// Check the comparison result and print the appropriate message
if (compareValue < 0)
{
// If the comparison value is less than 0, the specific date is in the past
System.Console.WriteLine("{0:d} is in the past.", theDay);
}
else if (compareValue == 0)
{
// If the comparison value is 0, the specific date is today
System.Console.WriteLine("{0:d} is today!", theDay);
}
else
{
// If the comparison value is greater than 0, the specific date has not come yet
System.Console.WriteLine("{0:d} has not come yet.", theDay);
}
}
}
Sample Output:
7/28/2017 has not come yet.
Flowchart :
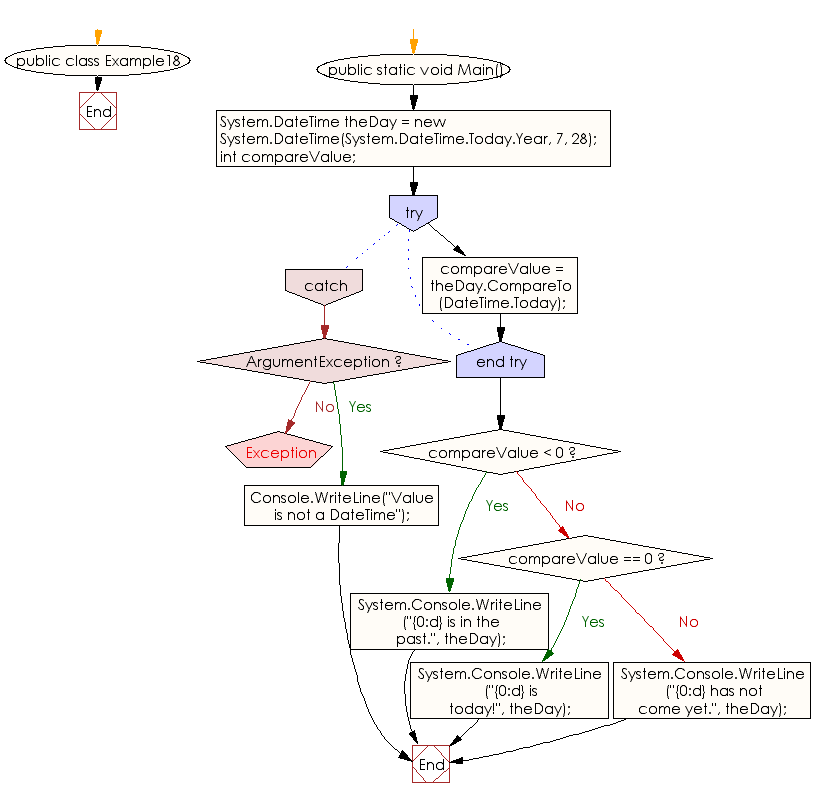
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a date one year previously and the date one year in the future compare to the current date.
Next: Write a C# Sharp program to get the number of days of the specified month and year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.