C#: Compare DateTime objects
Write a C# Sharp program to compare DateTime objects.
Sample Solution:-
C# Sharp Code:
using System;
public class Example20
{
public static void Main()
{
// Create some DateTime objects.
// Get the current Coordinated Universal Time (UTC) date and time
DateTime one = DateTime.UtcNow;
// Get the current local date and time
DateTime two = DateTime.Now;
// Assign the value of 'one' to 'three'
DateTime three = one;
// Compare the DateTime objects and display the results.
// Compare if 'one' is equal to 'two'
bool result = one.Equals(two);
Console.WriteLine("The result of comparing DateTime object one and two is: {0}.", result);
// Compare if 'one' is equal to 'three'
result = one.Equals(three);
Console.WriteLine("The result of comparing DateTime object one and three is: {0}.", result);
}
}
Sample Output:
The result of comparing DateTime object one and two is: False. The result of comparing DateTime object one and three is: True.
Flowchart :
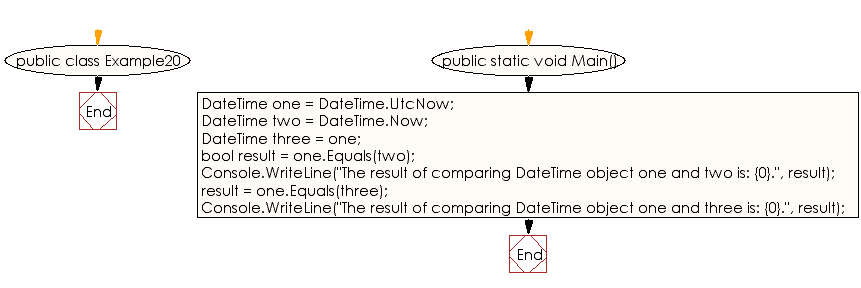
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get the number of days of the specified month and year.
Next: Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent using the specified array of formats, culture-specific format information, and style.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.