C#: Display short date format for the ja-JP culture
Write a C# Sharp program to display string representation of a date using the short date format specified for ja-JP culture.
Sample Solution:-
C# Sharp Code:
using System;
public class Example26
{
public static void Main(String[] argv)
{
// Create a DateTime object representing May 12, 2016, at 5:23:15 AM.
DateTime may12 = new DateTime(2016, 5, 12, 5, 23, 15, 16);
// Define the culture as "ja-JP" (Japanese - Japan).
IFormatProvider culture = new System.Globalization.CultureInfo("ja-JP", true);
// Get the short date formats using the "ja-JP" culture for the date 'may12'.
string[] frenchmay12Formats = may12.GetDateTimeFormats(culture);
// Display 'may12' in various formats using the "ja-JP" culture.
foreach (string format in frenchmay12Formats)
{
Console.WriteLine(format);
}
}
}
Sample Output:
2016/05/12 16/05/12 16/5/12 2016/5/12 2016-05-12 2016年5月12日 2016年05月12日 2016年5月12日 木曜日 2016年05月12日 木曜日 2016年5月12日 2016年5月12日 木曜日 2016年5月12日 2016年5月12日 木曜日 2016年5月12日 5:23 2016年5月12日 05:23 2016年5月12日 午前 5:23 ----- 2016年5月 2016年5月
Flowchart:
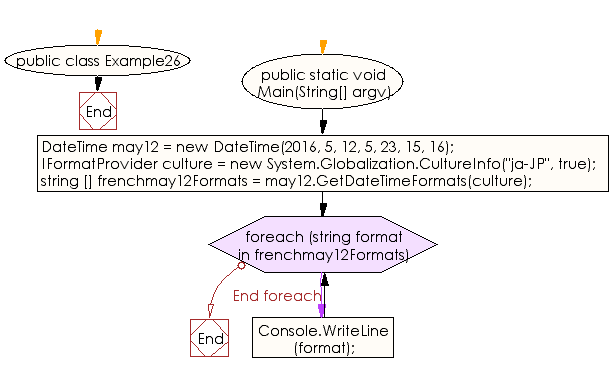
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the string representation of a date using the long date format.
Next: Write a C# Sharp program to determine the type of a particular object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.