C#: Convert the value of the current DateTime object to its equivalent long time string representation
Write a C# Sharp program to convert the current DateTime object value to its equivalent long time string representation.
Sample Solution:-
C# Sharp Code:
using System;
using System.Threading;
using System.Globalization;
public class Example33
{
public static void Main()
{
// Create an array of culture names.
String[] names = { "en-CA", "en-AU", "fr-CH", "de-AT" };
// Initialize a DateTime object.
DateTime dateValue = new System.DateTime(2016, 8, 17, 10, 30, 15);
// Iterate the array of culture names.
foreach (var name in names) {
// Change the culture of the current thread.
Thread.CurrentThread.CurrentCulture = CultureInfo.CreateSpecificCulture(name);
// Display the name of the current culture and the date.
Console.WriteLine("Current culture: {0}", CultureInfo.CurrentCulture.Name);
Console.WriteLine("Date: {0:G}", dateValue);
// Display the long time pattern and the long time.
Console.WriteLine("Long time pattern: '{0}'",
DateTimeFormatInfo.CurrentInfo.LongTimePattern);
Console.WriteLine("Long time with format string: {0:T}", dateValue);
Console.WriteLine("Long time with ToLongTimeString: {0}\n",
dateValue.ToLongTimeString());
}
}
}
Sample Output:
Current culture: en-CA Date: 2016-08-17 10:30:15 AM Long time pattern: 'h:mm:ss tt' Long time with format string: 10:30:15 AM Long time with ToLongTimeString: 10:30:15 AM Current culture: en-AU Date: 17/08/2016 10:30:15 AM Long time pattern: 'h:mm:ss tt' Long time with format string: 10:30:15 AM Long time with ToLongTimeString: 10:30:15 AM Current culture: fr-CH Date: 17.08.2016 10:30:15 Long time pattern: 'HH:mm:ss' Long time with format string: 10:30:15 Long time with ToLongTimeString: 10:30:15 Current culture: de-AT Date: 17.08.2016 10:30:15 Long time pattern: 'HH:mm:ss' Long time with format string: 10:30:15 Long time with ToLongTimeString: 10:30:15
Flowchart:
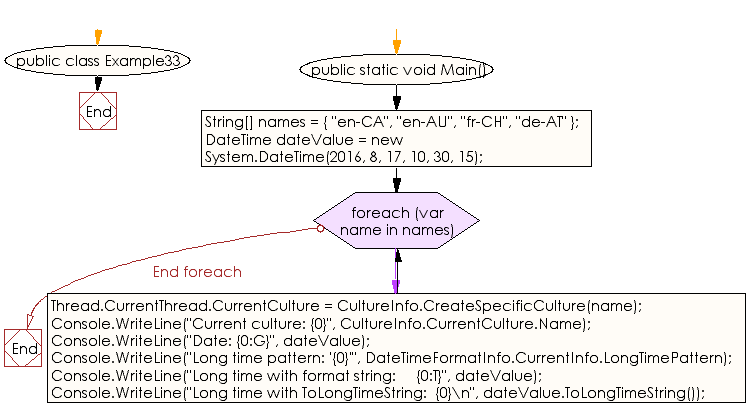
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent long date string representation.
Next: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent short date string representation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.