C#: Date and time value by using different conventions
Write a C# Sharp program to use these three format strings to display a date and time value. This is done by using the conventions of the en-CA and SV-FI cultures.
formats = { "G", "MM/yyyy", @"MM\/dd\/yyyy HH:mm", "yyyyMMdd" }
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
using System.Threading;
public class Example38
{
public static void Main()
{
// Array of date and time formats to display
String[] formats = { "G", "MM/yyyy", @"MM\/dd\/yyyy HH:mm", "yyyyMMdd" };
// Array of culture names
String[] cultureNames = { "en-CA", "sv-FI" };
// Specific DateTime object
DateTime date = new DateTime(2016, 5, 17, 13, 31, 17);
// Iterate through each culture
foreach (var cultureName in cultureNames) {
// Create a CultureInfo object for the specified culture
var culture = new CultureInfo(cultureName);
// Display the native name of the current culture
Console.WriteLine(culture.NativeName);
// Display the formatted date for each format in the formats array
foreach (var format in formats)
Console.WriteLine(" {0}: {1}", format, date.ToString(format));
Console.WriteLine(); // Add a line break between cultures for clarity
}
}
}
Sample Output:
English (Canada) G: 5/17/2016 1:31:17 PM MM/yyyy: 05/2016 MM\/dd\/yyyy HH:mm: 05/17/2016 13:31 yyyyMMdd: 20160517 svenska (Finland) G: 5/17/2016 1:31:17 PM MM/yyyy: 05/2016 MM\/dd\/yyyy HH:mm: 05/17/2016 13:31 yyyyMMdd: 20160517
Flowchart :
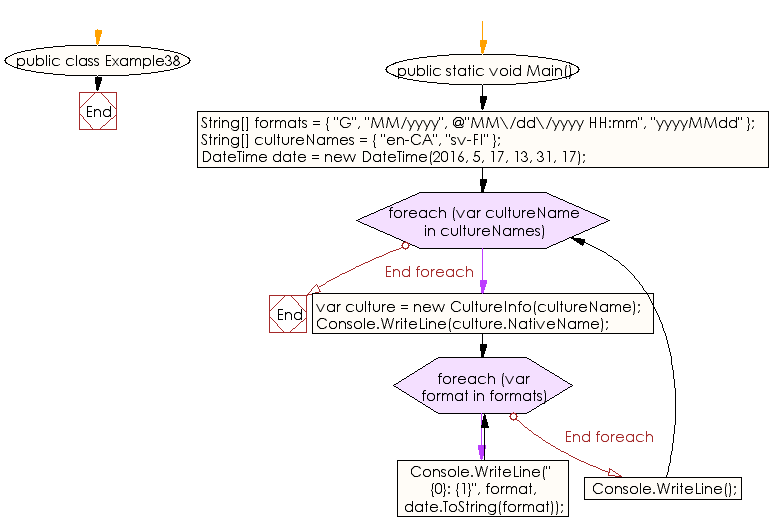
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the string representation of a date and time using CultureInfo objects that represent five different cultures.
Next: Write a C# Sharp program to use each of the standard date time format strings to display the string representation of a date and time for four different cultures.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.