C#: Get the last day of the current year against a given date
Write a program in C# Sharp to get the last day of the current year against a given date.
Sample Solution:-
C# Sharp Code:
using System;
class dttimeex47
{
static void Main()
{
int dt, mn, yr;
// Displaying the purpose of the program to the user.
Console.Write("\n\n Find the last day of a year against a date :\n");
Console.Write("---------------------------------------------------\n");
// Display the last day of the current year.
Console.WriteLine(" The Last day of the current year is : {0} \n", LastDayOfYear().ToString("dd/MM/yyyy"));
// Inputting a custom date from the user.
Console.Write(" Input the Day : ");
dt = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Month : ");
mn = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Year : ");
yr = Convert.ToInt32(Console.ReadLine());
// Creating a DateTime object with the provided day, month, and year.
DateTime d = new DateTime(yr, mn, dt);
Console.WriteLine(" The formatted Date is : {0}", d.ToString("dd/MM/yyyy"));
// Finding the last day of the year for the provided date.
DateTime nd = LastDayOfYear(d);
Console.WriteLine(" The Last day of the year {0} is : {1}\n", yr, nd.ToString("dd/MM/yyyy"));
}
// Method to find the last day of the year for the current date.
static DateTime LastDayOfYear()
{
return LastDayOfYear(DateTime.Today);
}
// Method to find the last day of the year for a given date.
static DateTime LastDayOfYear(DateTime d)
{
// Adding one year to the provided date and then subtracting one day gives the last day of the year.
DateTime nextYear = new DateTime(d.Year + 1, 1, 1);
return nextYear.AddDays(-1);
}
}
Sample Output:
Find the last day of a year against a date : --------------------------------------------------- The Last day of the current year is : 31/12/2017 Input the Day : 12 Input the Month : 06 Input the Year : 2017 The formatted Date is : 12/06/2017 The Last day of the year 2017 is : 31/12/2017
Flowchart :
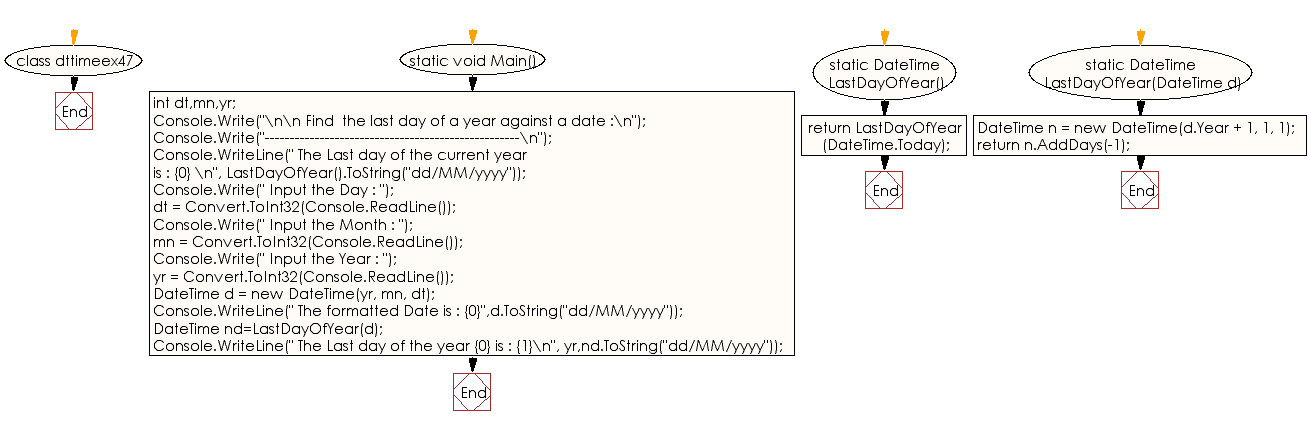
-->
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to get the first day of the current year and first of a year against a given date.
Next: Write a program in C# Sharp to get the number of days of a given month for a year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.