C#: Find the first day of a week against a given date
Write a program in C# Sharp to find the first day of a week against a given date.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
class dttimeex52 // Declaring a class named dttimeex52
{
static void Main() // Declaring the Main method
{
int yr, mn, dt; // Declaring variables for year, month, and day
Console.Write("\n\n Find the first day of a week against a given date :\n"); // Displaying a message in the console
Console.Write("--------------------------------------------------------\n"); // Displaying a separator line
// Input prompts for day, month, and year
Console.Write(" Input the Day : ");
dt = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Month : ");
mn = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Year : ");
yr = Convert.ToInt32(Console.ReadLine());
DateTime d = new DateTime(yr, mn, dt); // Creating a DateTime object with the provided year, month, and day
Console.WriteLine(" The formatted Date is : {0}", d.ToString("dd/MM/yyyy")); // Displaying the formatted input date
Console.WriteLine(" The first day of the week for the above date is : {0}\n", FirstDayOfWeek(d).ToString("dd/MM/yyyy")); // Displaying the first day of the week for the input date
}
// Method to find the first day of the week given a specific date
public static DateTime FirstDayOfWeek(DateTime dt)
{
var culture = System.Threading.Thread.CurrentThread.CurrentCulture; // Getting current culture information
var diff = dt.DayOfWeek - culture.DateTimeFormat.FirstDayOfWeek; // Calculating the difference between the input date's day of the week and the first day of the week in the culture
if (diff < 0)
diff += 7; // If the difference is negative, add 7 days to get the correct first day of the week
return dt.AddDays(-diff).Date; // Returning the date adjusted to the first day of the week
}
}
Sample Output:
Find the first day of a week against a given date : -------------------------------------------------------- Input the Day : 12 Input the Month : 06 Input the Year : 2017 The formatted Date is : 12/06/2017 The first day of the week for the above date is : 11/06/2017
Flowchart:
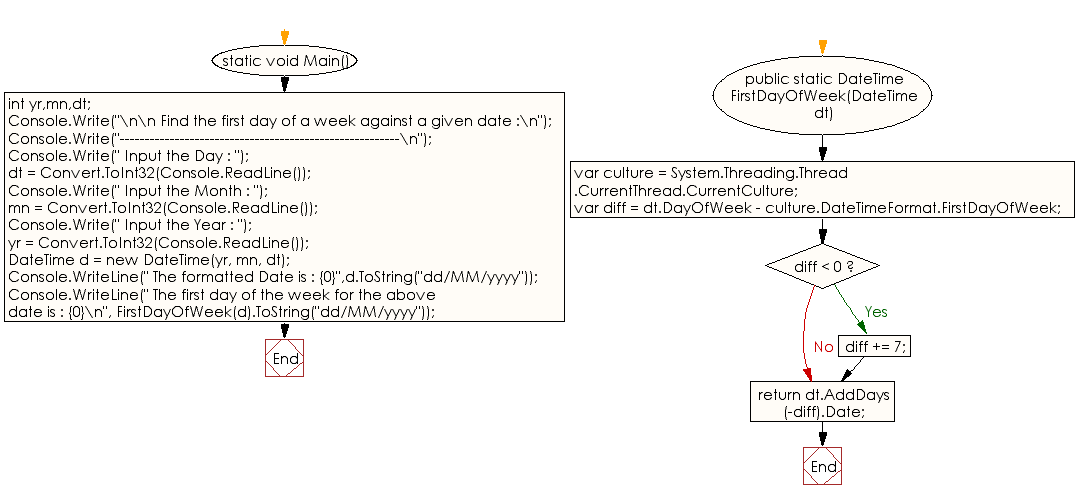
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to print the name of the first three letters of month of a year starting form current date.
Next: Write a program in C# Sharp to find the last day of a week against a given date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.