C#: Create a file with text and read the file
Write a C# Sharp program to create a text file and read it.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
using System.IO; // Importing the System.IO namespace for file operations
using System.Text; // Importing the System.Text namespace for encoding
class filexercise3 // Declaring a class named filexercise3
{
public static void Main() // Declaring the Main method
{
string fileName = @"mytest.txt"; // Initializing a string variable with the file name
try // Starting a try block to catch exceptions
{
// Delete the file if it exists.
if (File.Exists(fileName)) // Checking if the file exists
{
File.Delete(fileName); // If the file exists, delete it
}
// Displaying a message to create a file with text and read the file
Console.Write("\n\n Create a file with text and read the file :\n");
Console.Write("-------------------------------------------------\n");
// Create the file and write content to it.
using (StreamWriter fileStr = File.CreateText(fileName)) // Creating a StreamWriter to write text to the file
{
// Writing content to the file
fileStr.WriteLine(" Hello and Welcome");
fileStr.WriteLine(" It is the first content");
fileStr.WriteLine(" of the text file mytest.txt");
}
using (StreamReader sr = File.OpenText(fileName)) // Creating a StreamReader to read text from the file
{
string s = "";
Console.WriteLine(" Here is the content of the file mytest.txt : ");
// Reading and displaying content from the file
while ((s = sr.ReadLine()) != null) // Reading lines until the end of the file
{
Console.WriteLine(s); // Displaying each line in the console
}
Console.WriteLine(""); // Adding an empty line for better formatting
}
}
catch (Exception MyExcep) // Catching any exceptions that might occur
{
Console.WriteLine(MyExcep.ToString()); // Displaying the exception details if any
}
}
}
Sample Output:
Create a file with text and read the file : ------------------------------------------------- Here is the content of the file mytest.txt : Hello and Welcome It is the first content of the text file mytest.txt
Flowchart :
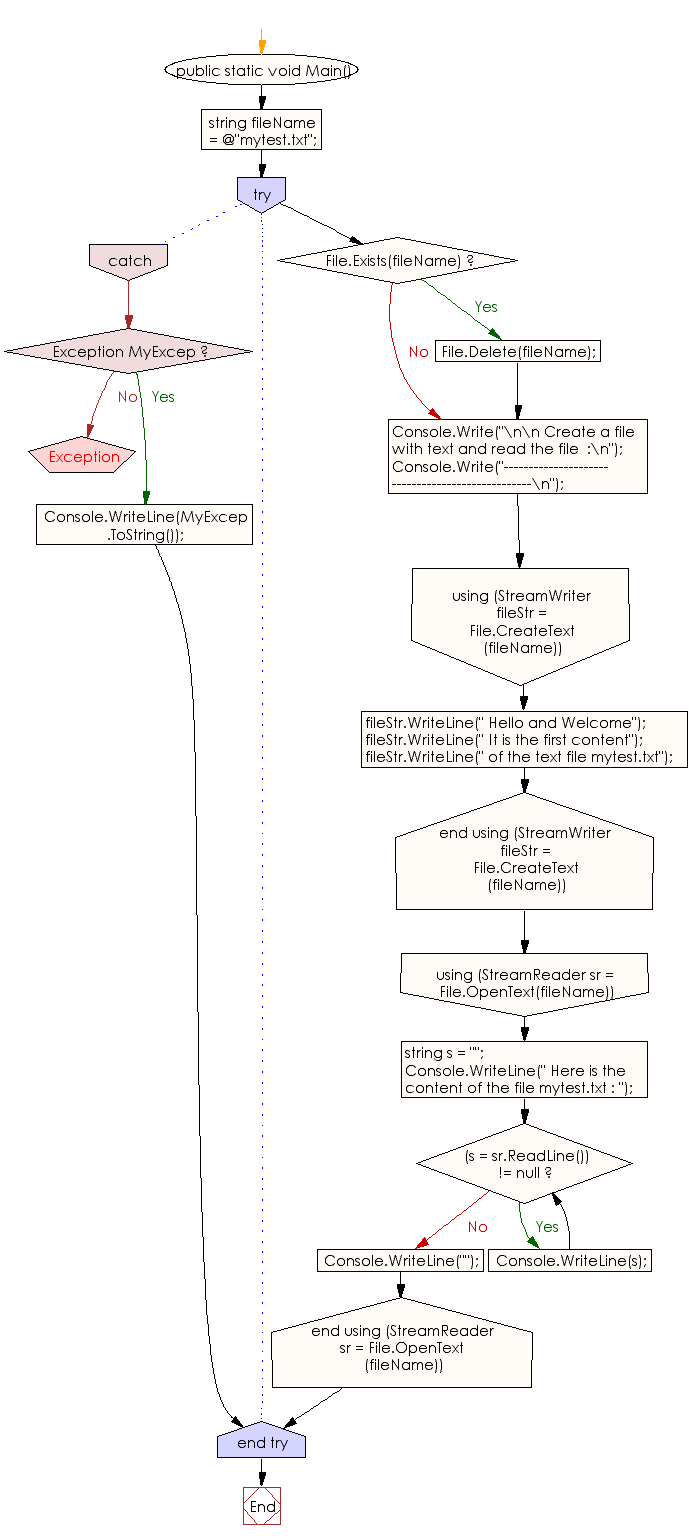
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a file and add some text.
Next: Write a program in C# Sharp to create a file and write an array of strings to the file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.