C#: Create a file and copy the file
Write a program in C# Sharp to create and copy the file to another name and display the content.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace for basic functionalities
using System.IO; // Importing the System.IO namespace for file operations
using System.Text; // Importing the System.Text namespace for encoding
public class SimpleFileCopy // Declaring a class named SimpleFileCopy
{
static void Main() // Declaring the Main method
{
string sfileName = @"mytest.txt"; // Initializing a string variable with the source file name
string tfileName = @"mynewtest.txt"; // Initializing a string variable with the target file name
// Delete the file if it exists.
if (File.Exists(sfileName)) // Checking if the source file exists and deleting it if true
{
File.Delete(sfileName); // Deleting the source file if it exists
}
// Displaying a message to create a file and write content to it
Console.Write("\n\n Create a file and copy the file :\n");
Console.Write("---------------------------------------\n");
// Create the source file and write initial content to it.
using (StreamWriter fileStr = File.CreateText(sfileName)) // Creating a StreamWriter to write text to the source file
{
// Writing initial content to the source file
fileStr.WriteLine(" Hello and Welcome");
fileStr.WriteLine(" It is the first content");
fileStr.WriteLine(" of the text file mytest.txt");
}
// Displaying the content of the source file
using (StreamReader sr = File.OpenText(sfileName)) // Opening a StreamReader to read the content of the source file
{
string s = "";
Console.WriteLine(" Here is the content of the file {0} : ", sfileName);
while ((s = sr.ReadLine()) != null) // Looping through each line until the end of the file
{
Console.WriteLine(s); // Displaying each line in the console
}
Console.WriteLine("");
}
// Copying the source file to a new target file
System.IO.File.Copy(sfileName, tfileName, true); // Copying the source file to the target file
// Displaying a message indicating successful copying
Console.WriteLine(" The file {0} successfully copied to the name {1} in the same directory.", sfileName, tfileName);
// Displaying the content of the target file
using (StreamReader sr = File.OpenText(tfileName)) // Opening a StreamReader to read the content of the target file
{
string s = "";
Console.WriteLine(" Here is the content of the file {0} : ", tfileName);
while ((s = sr.ReadLine()) != null) // Looping through each line until the end of the file
{
Console.WriteLine(s); // Displaying each line in the console
}
Console.WriteLine("");
}
Console.ReadKey(); // Waiting for a key press before exiting
}
}
Sample Output:
Create a file and copy the file : --------------------------------------- Here is the content of the file mytest.txt : Hello and Welcome It is the first content of the text file mytest.txt The file mytest.txt successfully copied to the name mynewtest.txt in the same directory. Here is the content of the file mynewtest.txt : Hello and Welcome It is the first content of the text file mytest.txt
Flowchart :
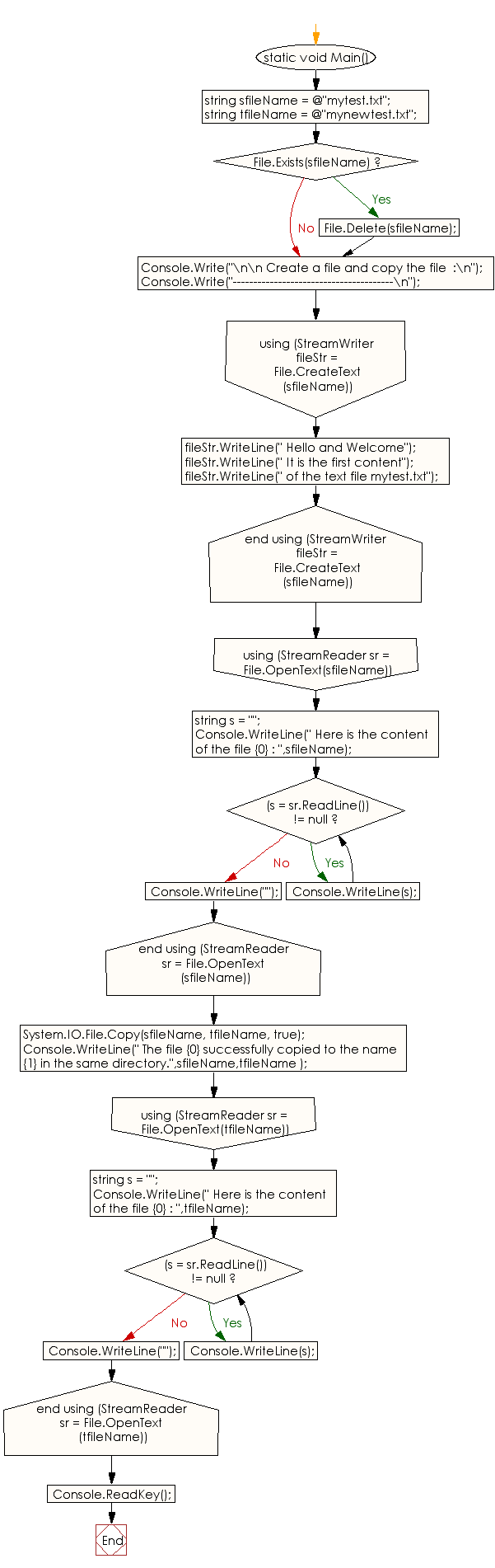
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to append some text to an existing file.
Next: Write a program in C# Sharp to create a file and move the file into the same directory to another name.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.