C#: Display the pattern like right angle triangle which repeat a number in a row
Write a program in C# Sharp to make such a pattern like a right angle triangle with a number which repeats a number in a row.
The pattern is as follows:
1 22 333 4444
Visual Presentation:
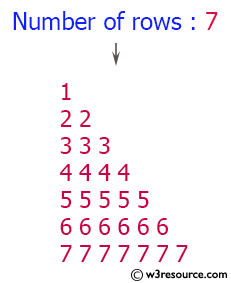
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise11 // Declaration of the Exercise11 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, rows; // Declaration of variables 'i', 'j' for iteration, 'rows' to store the number of rows
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like a right-angle triangle which repeats a number in a row:\n"); // Displaying the purpose of the program
Console.Write("-------------------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows : "); // Prompting the user to input the number of rows
rows = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
for (i = 1; i <= rows; i++) // Loop to iterate through each row
{
for (j = 1; j <= i; j++) // Loop to print the value of 'i' in each row 'i' times
{
Console.Write("{0}", i); // Displaying 'i' to form the pattern
}
Console.Write("\n"); // Moving to the next line to form the right-angled pattern
}
}
}
Sample Output:
Display the pattern like right angle triangle which repeat a number in a row: ------------------------------------------------------------------------------- Input number of rows : 7 1 22 333 4444 55555 666666 7777777
Flowchart:
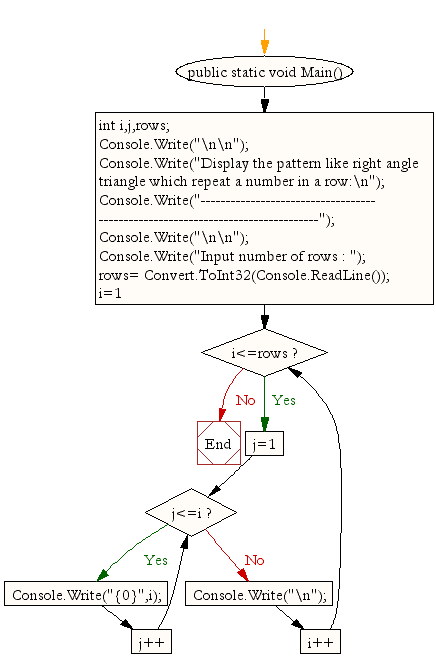
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the pattern like right angle triangle with a number.
Next: Write a program in C# Sharp to make such a pattern like right angle triangle with number increased by 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.