C#: Display the pattern like a pyramid containing an odd number of asterisks
C# Sharp For Loop: Exercise-20 with Solution
Write a program in C# Sharp to display the pattern like a pyramid using asterisk and each row contain an odd number of asterisks.
The pattern is as below:
* *** *****
Visual Presentation:
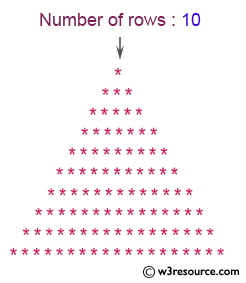
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise20 // Declaration of the Exercise20 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, n; // Declaration of variables of type integer
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like pyramid containing odd number of asterisks:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows for this pattern :"); // Prompting the user to input the number of rows for the pattern
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of rows entered by the user
// Loop to print the pattern
for (i = 0; i < n; i++)
{
for (j = 1; j <= n - i; j++) // Loop to print spaces before the asterisks
Console.Write(" ");
for (j = 1; j <= 2 * i - 1; j++) // Loop to print asterisks
Console.Write("*");
Console.Write("\n"); // Moving to the next line after printing each row of the pattern
}
}
}
Sample Output:
Display the pattern like pyramid containing odd number of asterisks: ---------------------------------------------------------------------- Input number of rows for this pattern :10 * *** ***** ******* ********* *********** ************* *************** *****************
Flowchart:
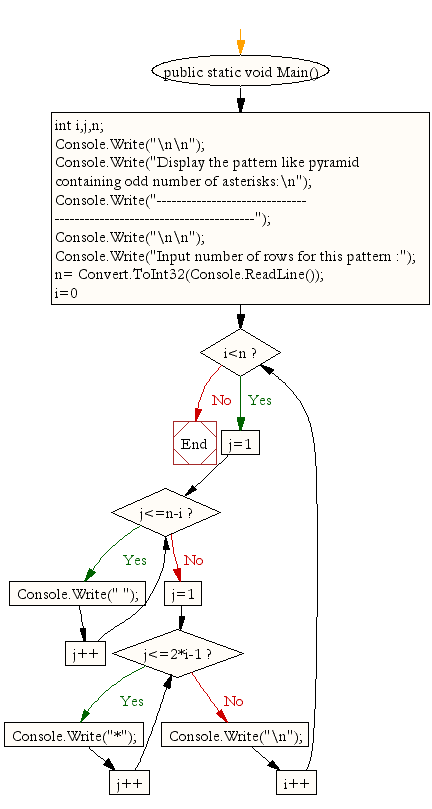
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to calculate the harmonic series and their sum.
Next: Write a program in C# Sharp to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics