C#: Print the Floyd's Triangle
Write a program in C# Sharp to print Floyd's Triangle. The Floyd's triangle is as below :
1 01 101 0101 10101
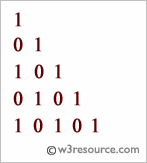
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise22 // Declaration of the Exercise22 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, n, p, q; // Declaration of variables i, j, n, p, q
Console.Write("\n\n"); // Displaying new lines
Console.Write("Print the Floyd's Triangle:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows : "); // Prompting the user to input the number of rows
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of rows entered by the user
// Loop to print the Floyd's Triangle pattern
for (i = 1; i <= n; i++)
{
// Setting p and q values based on whether i is even or odd
if (i % 2 == 0)
{
p = 1;
q = 0;
}
else
{
p = 0;
q = 1;
}
for (j = 1; j <= i; j++)
{
// Checking for even or odd position within the row to print p or q
if (j % 2 == 0)
Console.Write("{0}", p); // Printing p if j is even
else
Console.Write("{0}", q); // Printing q if j is odd
}
Console.Write("\n"); // Moving to the next line after printing each row
}
}
}
Sample Output:
Print the Floyd's Triangle: ----------------------------- Input number of rows : 8 1 01 101 0101 10101 010101 1010101 01010101
Flowchart:
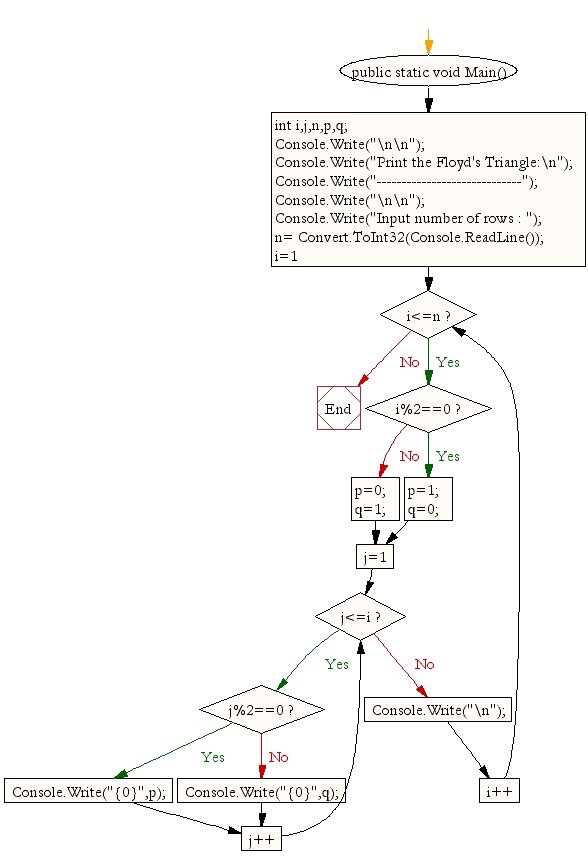
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
Next: Write a program in C# Sharp to display the sum of the series [ 1+x+x^2/2!+x^3/3!+....].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.