C#: Display the number in reverse order
C# Sharp For Loop: Exercise-37 with Solution
Write a program in C# Sharp to display a number in reverse order.
Visual Presentation:
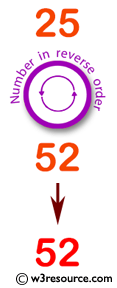
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise37 // Declaration of the Exercise37 class
{
public static void Main() // Main method, entry point of the program
{
int num, r, sum = 0, t; // Declaration of variables
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the number in reverse order:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input a number: "); // Prompting the user to input a number
num = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
for (t = num; t != 0; t = t / 10) // Loop to reverse the number
{
r = t % 10; // Extracting the last digit of the number
sum = sum * 10 + r; // Reversing the number by storing each digit sequentially
}
Console.Write("The number in reverse order is : {0} \n", sum); // Displaying the number in reverse order
}
}
Sample Output:
Display the number in reverse order: -------------------------------------- Input a number: 25 The number in reverse order is : 52
Flowchart:
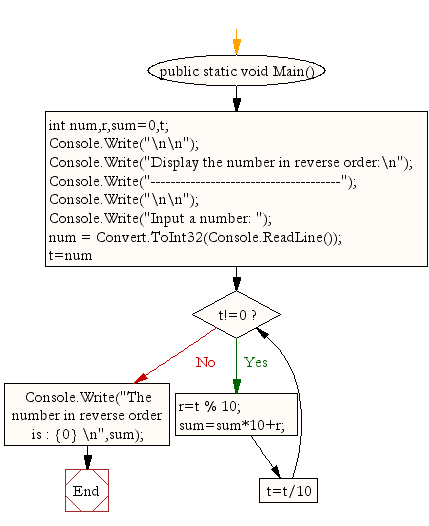
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the such a pattern for n number of rows using a number which will start with the number 1 and the first and a last number of each row will be 1.
Next: Write a program in C# Sharp to check whether a number is a palindrome or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-37.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics