C#: Read 10 numbers and find their sum and average
Write a C# Sharp program to read 10 numbers and find their average and sum.
Visual Presentation:
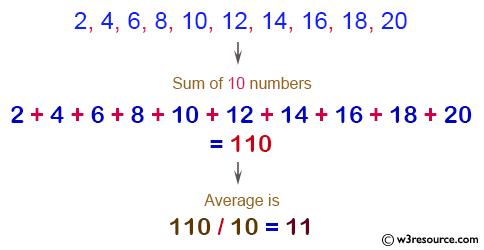
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise4 // Declaration of the Exercise4 class
{
public static void Main() // Main method, entry point of the program
{
int i, n, sum = 0; // Declaration of variables 'i' for iteration, 'n' to store each number, and 'sum' to store their total sum
double avg; // Declaration of variable 'avg' to store the average
Console.Write("\n\n"); // Displaying new lines
Console.Write("Read 10 numbers and calculate sum and average:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the 10 numbers : \n"); // Prompting the user to input 10 numbers
for (i = 1; i <= 10; i++) // Loop to read 10 numbers
{
Console.Write("Number-{0} :", i); // Prompting for each number with its respective index
n = Convert.ToInt32(Console.ReadLine()); // Reading each number entered by the user
sum += n; // Adding each number to the sum
}
avg = sum / 10.0; // Calculating the average by dividing the sum by 10 (the number of values)
Console.Write("The sum of 10 numbers is : {0}\nThe Average is : {1}\n", sum, avg); // Displaying the total sum and average of the 10 numbers
}
}
Sample Output:
Read 10 numbers and calculate sum and average: ---------------------------------------------- Input the 10 numbers : Number-1 :2 Number-2 :4 Number-3 :6 Number-4 :8 Number-5 :10 Number-6 :12 Number-7 :14 Number-8 :16 Number-9 :18 Number-10 :20 The sum of 10 no is : 110 The Average is : 11
Flowchart:
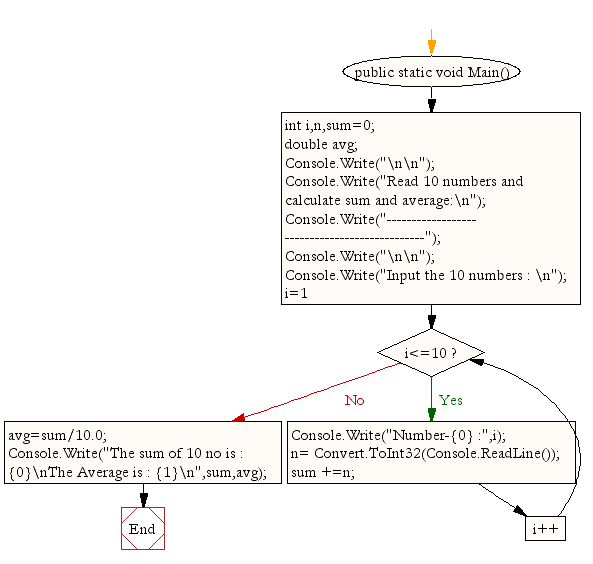
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display n terms of natural number and their sum.
Next: Write a program in C# Sharp to display the cube of the number upto given an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.