C#: Display the sum of n number of odd natural number
Write a C# Sharp program to display the n terms of odd natural numbers and their sums.
like: 1 3 5 7 ... n
Visual Presentation:
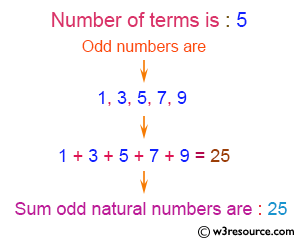
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise8 // Declaration of the Exercise8 class
{
public static void Main() // Main method, entry point of the program
{
int i, n, sum = 0; // Declaration of variables 'i' for iteration, 'n' to store the input number of terms, 'sum' to calculate the sum
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the sum of n odd natural numbers:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
Console.Write("\nThe odd numbers are :"); // Displaying the start of the sequence of odd numbers
for (i = 1; i <= n; i++) // Loop to generate 'n' odd natural numbers
{
Console.Write("{0} ", 2 * i - 1); // Displaying each odd number in the sequence
sum += 2 * i - 1; // Calculating the sum by adding each odd number
}
Console.Write("\nThe Sum of odd Natural Numbers up to {0} terms : {1} \n", n, sum); // Displaying the sum of the odd natural numbers up to 'n' terms
}
}
Sample Output:
Display the sum of n odd natural number: ------------------------------------------ Input number of terms : 5 The odd numbers are :1 3 5 7 9 The Sum of odd Natural Number upto 5 terms : 25
Flowchart:
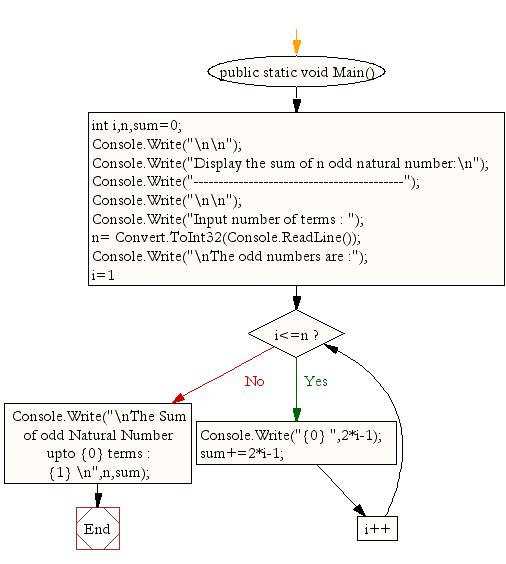
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the multiplication table vertically from 1 to n.
Next: Write a program in C# Sharp to display following Pattern.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.