C#: Display top nth records from the list
Write a program in C# Sharp to display the top nth records.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise11
{
static void Main(string[] args)
{
// Creating a list of integers named templist and initializing it with some values
List<int> templist = new List<int>();
templist.Add(5);
templist.Add(7);
templist.Add(13);
templist.Add(24);
templist.Add(6);
templist.Add(9);
templist.Add(8);
templist.Add(7);
// Displaying a message indicating the intention to display top 'n' records from the list
Console.Write("\nLINQ : Display top nth records from the list : ");
Console.Write("\n----------------------------------------------\n");
// Displaying the members of the list using a foreach loop
Console.WriteLine("\nThe members of the list are : ");
foreach (var lstnum in templist)
{
Console.WriteLine(lstnum + " ");
}
// Asking the user to input the number of records to display
Console.Write("How many records you want to display ? : ");
int n = Convert.ToInt32(Console.ReadLine()); // Accepting 'n' from the user
// Sorting the templist in ascending order and then reversing it to get descending order
templist.Sort();
templist.Reverse();
// Displaying the top 'n' records from the sorted list using Take method
Console.Write("The top {0} records from the list are : \n", n);
foreach (int topn in templist.Take(n))
{
Console.WriteLine(topn);
}
}
}
Sample Output:
---------------------------------------------- The members of the list are : 5 7 13 24 6 9 8 7 How many records you want to display ? : 5 The top 5 records from the list are : 24 13 9 8 7
Visual Presentation:
Flowchart:
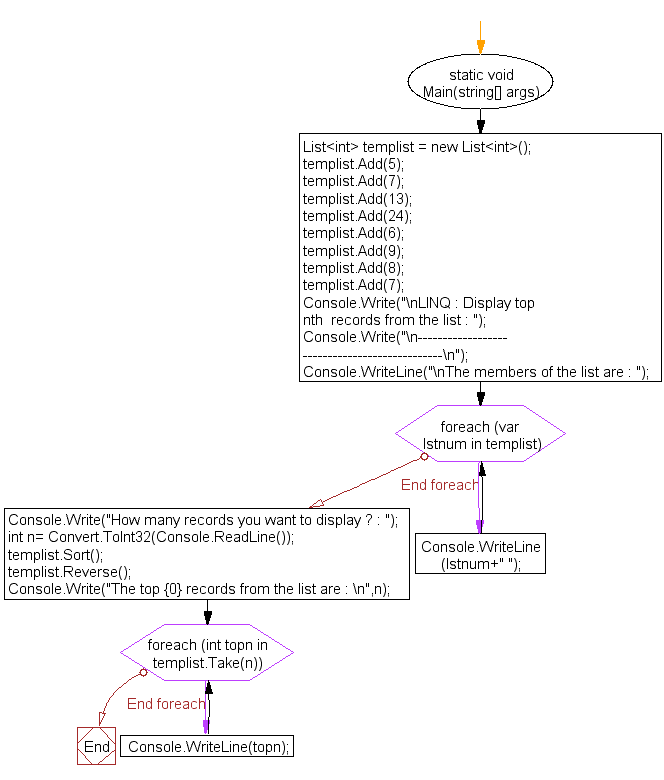
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to Accept the members of a list through the keyboard and display the members more than a specific value.
Next: Write a program in C# Sharp to find the uppercase words in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.