C#: Find the nth Maximum Grade Point achieved by the students from the list of student
Write a program in C# Sharp to find the n-th maximum grade point achieved by the students from the list of students.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
// Define a class named Students
public class Students
{
// Properties of the Students class: StuName, GrPoint, StuId
public string StuName { get; set; }
public int GrPoint { get; set; }
public int StuId { get; set; }
// Method to get student records
public List<Students> GtStuRec()
{
// Create a list of Students and add student records to it
List<Students> stulist = new List<Students>();
stulist.Add(new Students { StuId = 1, StuName = " Joseph ", GrPoint = 800 });
// ... (similar lines for other students)
return stulist; // Return the list of student records
}
}
// Define a class named LinqExercise14
class LinqExercise14
{
// Main method, the entry point of the program
static void Main(string[] args)
{
Students e = new Students(); // Create an instance of the Students class
// Display information about the program
Console.Write("\nLINQ : Find the nth Maximum Grade Point achieved by the students from the list of student : ");
Console.Write("\n------------------------------------------------------------------------------------------\n");
// Ask the user for the rank of the maximum grade point to find
Console.Write("Which maximum grade point(1st, 2nd, 3rd, ...) you want to find : ");
int grPointRank = Convert.ToInt32(Console.ReadLine());
Console.Write("\n");
var stulist = e.GtStuRec(); // Get the list of student records
// Perform LINQ query to group student records by Grade Point and order them in descending order
var students = (from stuMast in stulist
group stuMast by stuMast.GrPoint into g
orderby g.Key descending
select new
{
StuRecord = g.ToList()
}).ToList();
// Display the student record with the specified nth maximum grade point
students[grPointRank - 1].StuRecord
.ForEach(i => Console.WriteLine(" Id : {0}, Name : {1}, achieved Grade Point : {2}", i.StuId, i.StuName, i.GrPoint));
Console.ReadKey(); // Wait for user input before closing the program
}
}
Sample Output:
LINQ : Find the nth Maximum Grade Point achieved by the students fro m the list of student : -------------------------------------------------------------------- ---------------------- Which maximum grade point(1st, 2nd, 3rd, ...) you want to find : 3 Id : 7, Name : David, achieved Grade Point : 750 Id : 10, Name : Jenny, achieved Grade Point : 750
Flowchart:
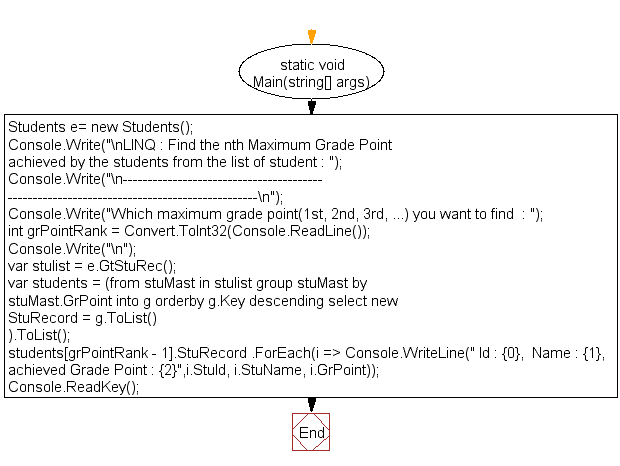
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a string array to a string.
Next: Write a program in C# Program to Count File Extensions and Group it using LINQ.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.