C#: Generate a cartesian product of two sets
C# Sharp LINQ : Exercise-23 with Solution
Write a program in C# Sharp to generate a cartesian product of two sets.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise23
{
public static void Main(string[] args)
{
// Define two sets: charset1 and numset1
char[] charset1 = { 'X', 'Y', 'Z' };
int[] numset1 = { 1, 2, 3, 4 };
// Display a message indicating the purpose of the following output
Console.Write("\nLINQ : Generate a cartesian product of two sets : ");
Console.Write("\n------------------------------------------------\n");
// Use LINQ to generate the Cartesian product of the two sets
var cartesianProduct = from letterList in charset1
from numberList in numset1
select new { letterList, numberList };
// Display the Cartesian product generated
Console.Write("The cartesian product are : \n");
foreach (var productItem in cartesianProduct)
{
Console.WriteLine(productItem);
}
Console.ReadLine(); // Wait for user input before exiting
}
}
OR
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise23
{
public static void Main(string[] args)
{
var set1 = new string[] {"X", "Y", "Z"};
var set2 = new int[] {1, 2, 3};
Console.Write("\nLINQ : Generate a cartesian product of two sets : ");
Console.Write("\n------------------------------------------------\n");
var cartesianProduct=
set1.SelectMany(p=> set2.Select(q=> p+q+' '));
Console.Write("The cartesian product are : \n");
foreach (var ProductList in cartesianProduct)
{
Console.Write(ProductList);
}
Console.ReadLine();
}
}
Sample Output:
LINQ : Generate a cartesian product of two sets : ------------------------------------------------ The Cartesian Product are : { letterList = X, numberList = 1 } { letterList = X, numberList = 2 } { letterList = X, numberList = 3 } { letterList = X, numberList = 4 } { letterList = Y, numberList = 1 } { letterList = Y, numberList = 2 } { letterList = Y, numberList = 3 } { letterList = Y, numberList = 4 } { letterList = Z, numberList = 1 } { letterList = Z, numberList = 2 } { letterList = Z, numberList = 3 } { letterList = Z, numberList = 4 }
Visual Presentation:
Flowchart:
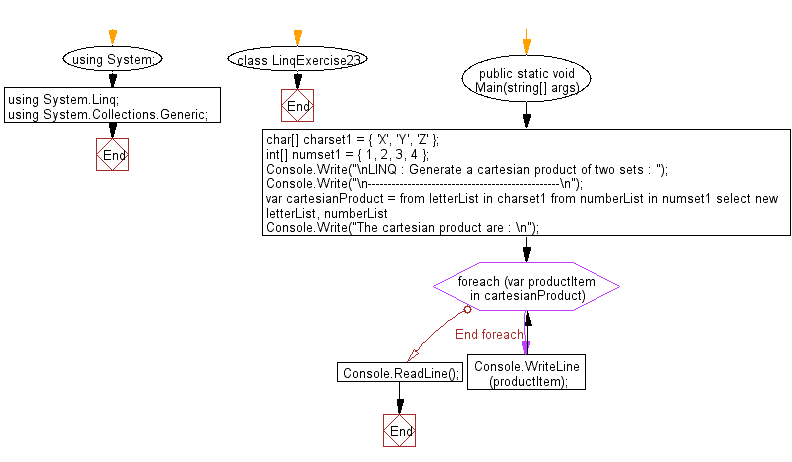
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the strings for a specific minimum length.
Next: Write a program in C# Sharp to generate a Cartesian Product of three sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics