C#: Display the list according to the length then by name in ascending order
Write a program in C# Sharp to display the list of items in the array according to the length of the string then by name in ascending order.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise28
{
static void Main(string[] args)
{
// Array of cities
string[] cities =
{
"ROME","LONDON","NAIROBI","CALIFORNIA","ZURICH","NEW DELHI","AMSTERDAM","ABU DHABI", "PARIS"
};
// Displaying the instruction for the operation
Console.Write("\nLINQ : Display the list according to the length then by name in ascending order : ");
Console.Write("\n--------------------------------------------------------------------------------\n");
Console.Write("\nThe cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI','AMSTERDAM','ABU DHABI','PARIS' \n");
Console.Write("\nHere is the arranged list :\n");
// Ordering the cities first by length and then by name in ascending order using LINQ
IEnumerable<string> cityOrder =
cities.OrderBy(str => str.Length) // Order the cities by their lengths
.ThenBy(str => str); // Then order them alphabetically if lengths are equal
// Displaying the sorted cities
foreach (string city in cityOrder)
{
Console.WriteLine(city);
}
Console.ReadLine(); // Wait for user input before closing the console
}
}
Sample Output:
LINQ : Display the list according to the length then by name in ascending order : -------------------------------------------------------------------------------- The cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI','AMSTERDAM','ABU DHABI','PARIS' Here is the arranged list : ROME PARIS LONDON ZURICH NAIROBI ABU DHABI AMSTERDAM NEW DELHI CALIFORNIA
Visual Presentation:
Flowchart:
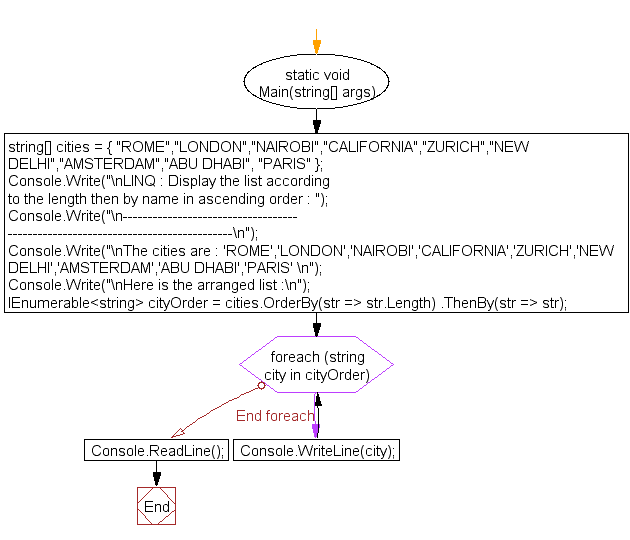
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to generate a Right Outer Join between two data sets.
Next: Write a program in C# Sharp to split a collection of strings into some groups.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.