C#: Arrange distinct elements in the list in ascending order
C# Sharp LINQ : Exercise-30 with Solution
Write a program in C# Sharp to arrange the distinct elements in the list in ascending order.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise30
{
static void Main(string[] args)
{
// Displaying the instruction for the operation
Console.Write("\nLINQ : Arrange distinct elements in the list in ascending order : ");
Console.Write("\n----------------------------------------------------------------\n");
// Retrieving the item descriptions from the GetItemMast method and arranging distinct elements in ascending order
var itemlist = (from c in Item_Mast.GetItemMast()
select c.ItemDes)
.Distinct() // Getting distinct item descriptions
.OrderBy(x => x); // Sorting the distinct item descriptions in ascending order
// Displaying the arranged distinct elements
foreach (var item in itemlist)
{
Console.WriteLine(item);
}
Console.ReadLine(); // Wait for user input before closing the console
}
}
class Item_Mast
{
public int ItemId { get; set; }
public string ItemDes { get; set; }
// Method to retrieve a list of Item_Mast objects
public static List<Item_Mast> GetItemMast()
{
// Creating and returning a list of Item_Mast objects with sample data
List<Item_Mast> itemlist = new List<Item_Mast>();
itemlist.Add(new Item_Mast() { ItemId = 1, ItemDes = "Biscuit " });
itemlist.Add(new Item_Mast() { ItemId = 2, ItemDes = "Honey " });
itemlist.Add(new Item_Mast() { ItemId = 3, ItemDes = "Butter " });
itemlist.Add(new Item_Mast() { ItemId = 4, ItemDes = "Brade " });
itemlist.Add(new Item_Mast() { ItemId = 5, ItemDes = "Honey " });
itemlist.Add(new Item_Mast() { ItemId = 6, ItemDes = "Biscuit " });
return itemlist;
}
}
Sample Output:
LINQ : Arrange distinct elements in the list in ascending order : ---------------------------------------------------------------- Biscuit Brade Butter Honey
Flowchart:
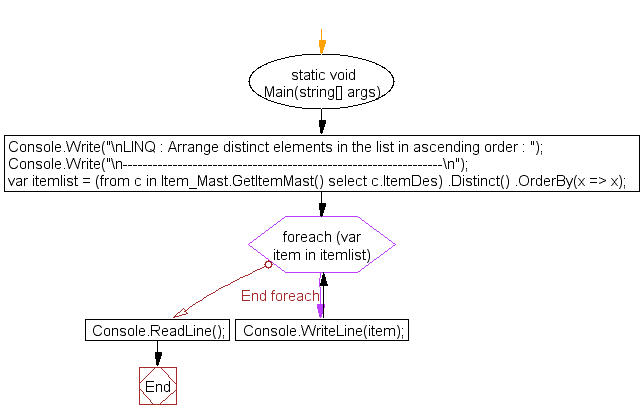
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to split a collection of strings into some groups.
Next: C# Sharp STRUCTURE Exercises.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/linq/csharp-linq-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics