C#: Display the number and frequency of number from given array
C# Sharp LINQ : Exercise-4 with Solution
Write a program in C# Sharp to display the number and frequency of a given number from an array.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise4
{
static void Main(string[] args)
{
// Define an integer array
int[] arr1 = new int[] { 5, 9, 1, 2, 3, 7, 5, 6, 7, 3, 7, 6, 8, 5, 4, 9, 6, 2 };
// Display a message indicating the purpose of the LINQ operation
Console.Write("\nLINQ : Display the number and frequency of number from given array : \n");
Console.Write("---------------------------------------------------------------------\n");
Console.Write("The numbers in the array are : \n");
Console.Write(" 5, 9, 1, 2, 3, 7, 5, 6, 7, 3, 7, 6, 8, 5, 4, 9, 6, 2\n");
// Group the array elements by their values and count occurrences
var n = from x in arr1
group x by x into y
select y;
// Display the number and its frequency of occurrence
Console.WriteLine("\nThe number and the Frequency are : \n");
foreach (var arrNo in n)
{
Console.WriteLine("Number " + arrNo.Key + " appears " + arrNo.Count() + " times");
}
Console.WriteLine("\n"); // Add newline for formatting
}
}
Sample Output:
LINQ : Display the number and frequency of number from given array : --------------------------------------------------------------------- The numbers in the array are : 5, 9, 1, 2, 3, 7, 5, 6, 7, 3, 7, 6, 8, 5, 4, 9, 6, 2 The number and the Frequency are : Number 5 appears 3 times Number 9 appears 2 times Number 1 appears 1 times Number 2 appears 2 times Number 3 appears 2 times Number 7 appears 3 times Number 6 appears 3 times Number 8 appears 1 times Number 4 appears 1 times
Visual Presentation:
Flowchart:
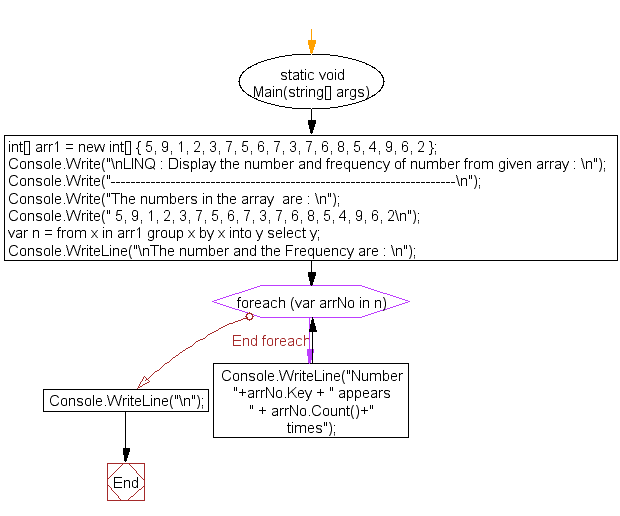
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the number of an array and the square of each number which is more than 20.
Next: Write a program in C# Sharp to display the characters and frequency of character from giving string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/linq/csharp-linq-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics