C#: Display the name of the days of a week
C# Sharp LINQ : Exercise-6 with Solution
Write a program in C# Sharp to display the name of the days of the week.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise6
{
static void Main(string[] args)
{
// Define an array containing the days of the week
string[] dayWeek = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" };
// Display a message indicating the purpose of the LINQ operation
Console.Write("\nLINQ : Display the name of the days of a week : ");
Console.Write("\n------------------------------------------------\n");
// Select all the days of the week using LINQ
var days = from WeekDay in dayWeek
select WeekDay;
// Display the days of the week
foreach (var WeekDay in days)
{
Console.WriteLine(WeekDay);
}
Console.WriteLine(); // Add a newline for formatting
}
}
Sample Output:
LINQ : Display the name of the days of a week : ------------------------------------------------ Sunday Monday Tuesday Wednesday Thursday Friday Saturday
Visual Presentation:
Flowchart:
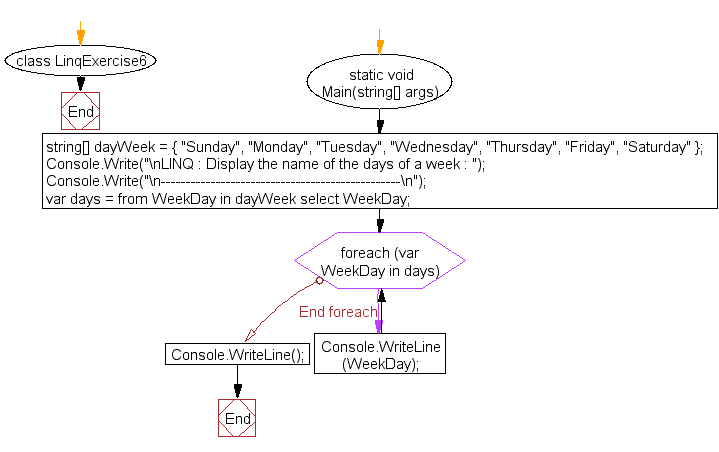
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the characters and frequency of character from giving string.
Next: Write a program in C# Sharp to display numbers, multiplication of number with frequency and the frequency of number of giving array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/linq/csharp-linq-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics