C#: Absolute value of a number of Decimal values, Double values, Int16 values, Int32 values: and Int64 values
C# Sharp Math: Exercise-1 with Solution
Write a C# Sharp program to get the absolute value of a number of Decimal values, Double values, Int16 values, Int32 values and Int64 values.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises {
class Program {
static void Main(string[] args) {
decimal[] decimals = {
Decimal.MaxValue,
15.55M,
0M,
-17.23M,
Decimal.MinValue
};
Console.WriteLine("Absolute value of a number of Decimal values:");
foreach(decimal value in decimals)
Console.WriteLine($"Abs({value}) = {Math.Abs(value)}");
double[] doubles = {
Double.MaxValue,
15.354e-17,
14.098123,
0,
-17.069713,
-14.058e18,
Double.MinValue
};
Console.WriteLine("Absolute value of a number of Double values:");
foreach(double value in doubles)
Console.WriteLine($"Abs({value}) = {Math.Abs(value)}");
short[] values1 = {
Int16.MaxValue,
10328,
0,
-1476,
Int16.MinValue
};
Console.WriteLine("Absolute value of a number of Int16 values:");
foreach(short value in values1) {
try {
Console.WriteLine($"Abs({value}) = {Math.Abs(value)}");
} catch (OverflowException) {
Console.WriteLine("Unable to calculate the absolute value of {0}.",
value);
}
}
Console.WriteLine("Absolute value of a number of Int32 values:");
int[] values2 = {
Int32.MaxValue,
16921,
0,
-804128,
Int32.MinValue
};
foreach(int value in values2) {
try {
Console.WriteLine($"Abs({value}) = {Math.Abs(value)}");
} catch (OverflowException) {
Console.WriteLine("Unable to calculate the absolute value of {0}.",
value);
}
}
long[] values3 = {
Int64.MaxValue,
109013,
0,
-6871982,
Int64.MinValue
};
Console.WriteLine("Absolute value of a number of Int64 values:");
foreach(long value in values3) {
try {
Console.WriteLine($"Abs({value}) = {Math.Abs(value)}");
} catch (OverflowException) {
Console.WriteLine("Unable to calculate the absolute value of {0}.",
value);
}
}
}
}
}
Sample Output:
Absolute value of a number of Decimal values: Abs(79228162514264337593543950335) = 79228162514264337593543950335 Abs(15.55) = 15.55 Abs(0) = 0 Abs(-17.23) = 17.23 Abs(-79228162514264337593543950335) = 79228162514264337593543950335 Absolute value of a number of Double values: Abs(1.79769313486232E+308) = 1.79769313486232E+308 Abs(1.5354E-16) = 1.5354E-16 Abs(14.098123) = 14.098123 Abs(0) = 0 Abs(-17.069713) = 17.069713 Abs(-1.4058E+19) = 1.4058E+19 Abs(-1.79769313486232E+308) = 1.79769313486232E+308 Absolute value of a number of Int16 values: Abs(32767) = 32767 Abs(10328) = 10328 Abs(0) = 0 Abs(-1476) = 1476 Unable to calculate the absolute value of -32768. Absolute value of a number of Int32 values: Abs(2147483647) = 2147483647 Abs(16921) = 16921 Abs(0) = 0 Abs(-804128) = 804128 Unable to calculate the absolute value of -2147483648. Absolute value of a number of Int64 values: Abs(9223372036854775807) = 9223372036854775807 Abs(109013) = 109013 Abs(0) = 0 Abs(-6871982) = 6871982 Unable to calculate the absolute value of -9223372036854775808.
Flowchart:
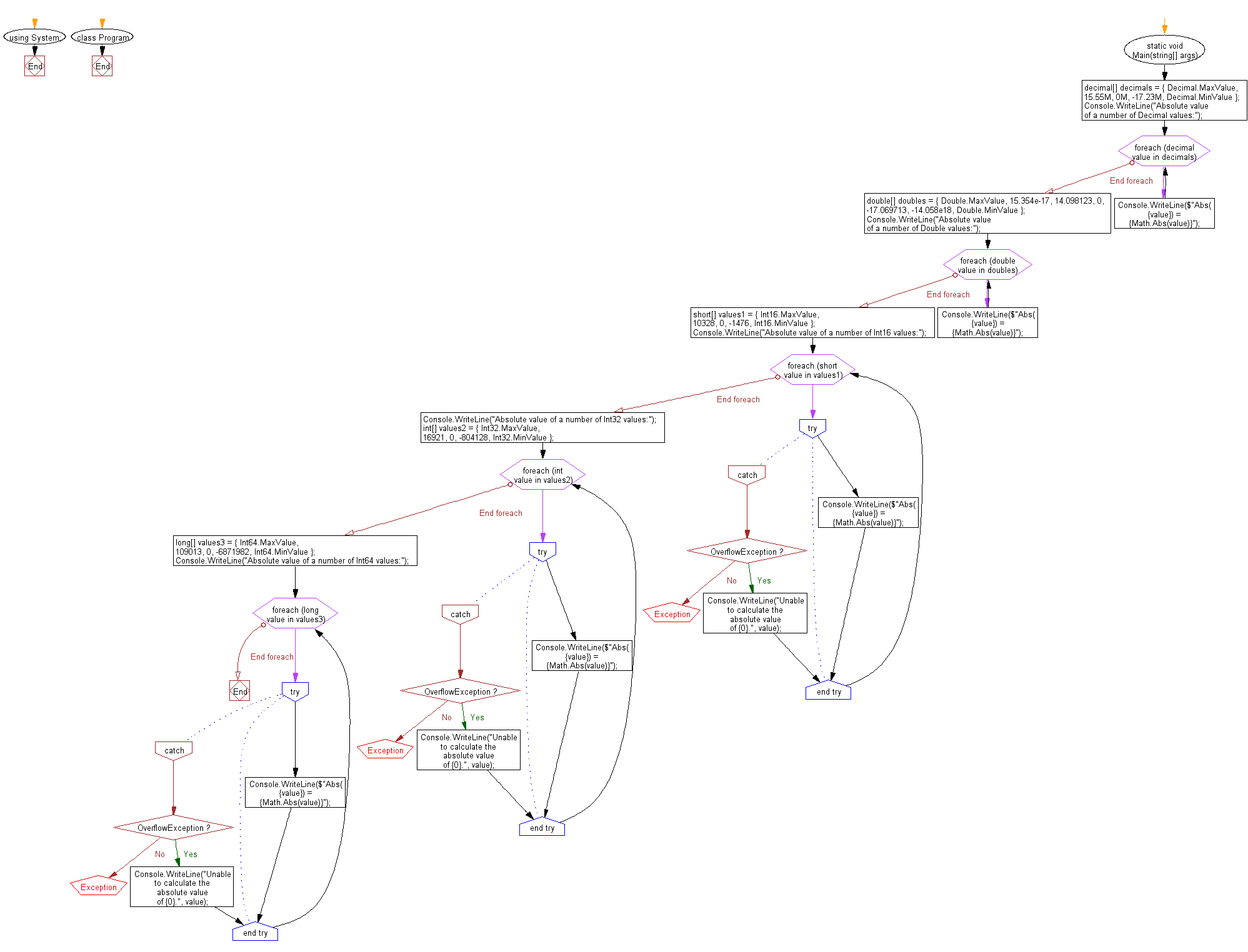
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: C# Sharp Math Exercises.
Next: Write a C# Sharp program to find the greater and smaller value of two variables.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/math/csharp-math-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics