C#: Check a string is Valid Hex Code or not
C# Sharp Regular Expression: Exercise-1 with Solution
Hex color codes start with a pound sign or hashtag (#) and are followed by six letters (A-F) and/or number (digit from 0-9). Alphabetic characters may be uppercase or lowercase.
Write a C# Sharp program to check whether a given string is a valid Hex code or not. Return true if this string is a valid code otherwise false.
Sample Data:
("#CD5C5C") -> True
("#f08080") -> True
("#E9967A") -> True
("#EFFA07A") -> False
Sample Solution-1:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with a hexadecimal color code
string hc = "#CD5C5C";
// Display the hexadecimal code
Console.WriteLine("Hex Code: " + hc);
// Check if the string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with a new hexadecimal color code
hc = "#f08080";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with another hexadecimal color code
hc = "#E9967A";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with another hexadecimal color code (with a mistake)
hc = "#EFFA07A";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
}
// Method to test if a string represents a valid hexadecimal color code
public static bool test(string hc)
{
// Use Regex to check if the string matches the pattern for a valid hex code
return Regex.IsMatch(hc, @"[#][0-9A-Fa-f]{6}\b");
}
}
}
Sample Output:
Hex Code: #CD5C5C Check the said string is valid hex code or not: True Hex Code: #f08080 Check the said string is valid hex code or not: True Hex Code: #E9967A Check the said string is valid hex code or not: True Hex Code: #EFFA07A Check the said string is valid hex code or not: False
Flowchart:
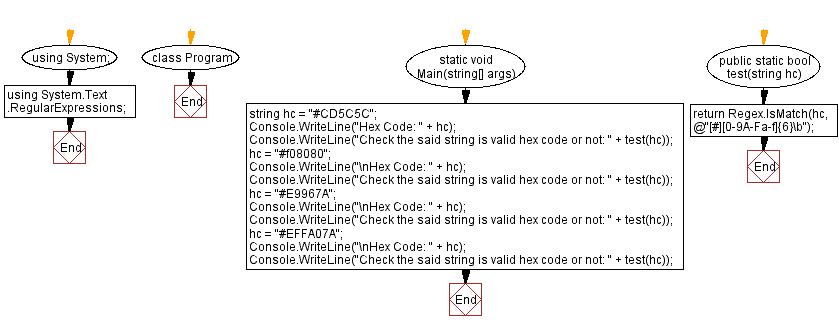
Sample Solution-2:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with a hexadecimal color code
string hc = "#CD5C5C";
// Display the hexadecimal code
Console.WriteLine("Hex Code: " + hc);
// Check if the string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with a new hexadecimal color code
hc = "#f08080";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with another hexadecimal color code
hc = "#E9967A";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
// Update the string variable with another hexadecimal color code (with a mistake)
hc = "#EFFA07A";
// Display the new hexadecimal code
Console.WriteLine("\nHex Code: " + hc);
// Check if the new string is a valid hex code and display the result
Console.WriteLine("Check the said string is valid hex code or not: " + test(hc));
}
// Method to test if a string represents a valid hexadecimal color code
public static bool test(string hc)
{
// Use Regex to match the string against the pattern for a valid hex code
// The pattern checks for a '#' followed by exactly 6 characters from 0-9 or a-f (case-insensitive)
// The "^" and "$" ensure the string matches the entire pattern from start to end
return Regex.Match(hc, "^#[0-9a-fA-F]{6}$").Success;
}
}
}
Sample Output:
Hex Code: #CD5C5C Check the said string is valid hex code or not: True Hex Code: #f08080 Check the said string is valid hex code or not: True Hex Code: #E9967A Check the said string is valid hex code or not: True Hex Code: #EFFA07A Check the said string is valid hex code or not: False
Flowchart:
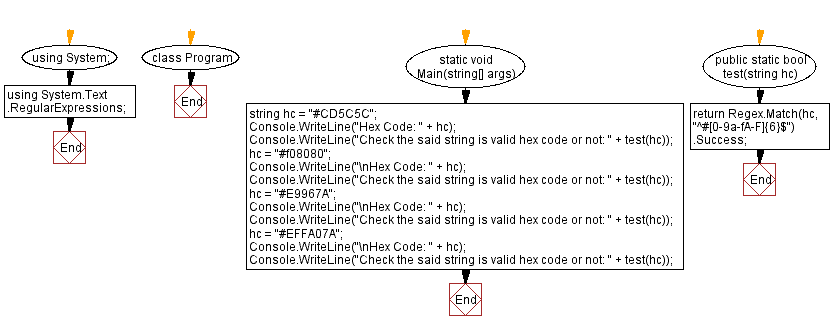
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: C# Sharp Regular Expression Exercises.
Next C# Sharp Exercise: Calculate the average word length in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics