C#: Validate a password
Write a C# Sharp program to validate a password of length 7 to 16 characters with the following guidelines:
• Length between 7 and 16 characters.
• At least one lowercase letter (a-z).
• At least one uppercase letter (A-Z).
• At least one digit (0-9).
• Supported special characters: ! @ # $ % ^ & * ( ) + = _ - { } [ ] : ; " ' ? < > , .
Sample Data:
("Suuu$21g@") -> True
("W#1g@") -> False
("a&&g@") -> False
("sdsd723#$Amid") -> True
("sdsd723#$Amidkiouy") -> False
Sample Solution:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with a password-like content
string text = "Suuu$21g@";
// Display the original string
Console.WriteLine("Original string: " + text);
// Check if the string is a valid password
Console.WriteLine("Check the said string is a valid password? " + test(text));
// Update the string variable with a different content
text = "W#1g@";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the updated string is a valid password
Console.WriteLine("Check the said string is a valid password? " + test(text));
// Update the string variable with another content
text = "a&&g@";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the updated string is a valid password
Console.WriteLine("Check the said string is a valid password? " + test(text));
// Update the string variable with more content
text = "sdsd723#$Amid";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the updated string is a valid password
Console.WriteLine("Check the said string is a valid password? " + test(text));
// Update the string variable with even more content
text = "sdsd723#$Amidkiouy";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the updated string is a valid password
Console.WriteLine("Check the said string is a valid password? " + test(text));
}
// Method to check if a string is a valid password
public static bool test(string text)
{
// Check if the length of the string is between 7 and 16 characters
// Check if the string contains at least one uppercase letter, one lowercase letter, one digit, and one special character
// Ensure that the string doesn't contain any character that doesn't belong to the allowed set
bool result = text.Length >= 7 && text.Length <= 16
&& Regex.IsMatch(text, "[A-Z]")
&& Regex.IsMatch(text, "[a-z]")
&& Regex.IsMatch(text, @"\d")
&& Regex.IsMatch(text, @"[!-/:-@\[-_{-~]")
&& !Regex.IsMatch(text, @"[^\dA-Za-z!-/:-@\[-_{-~]");
// Return the result indicating whether the string is a valid password
return result;
}
}
}
Sample Output:
Original string: Suuu$21g@ Check the said string is a valid passward? True Original string: W#1g@ Check the said string is a valid passward? False Original string: a&&g@ Check the said string is a valid passward? False Original string: sdsd723#$Amid Check the said string is a valid passward? True Original string: sdsd723#$Amidkiouy Check the said string is a valid passward? False
Flowchart:
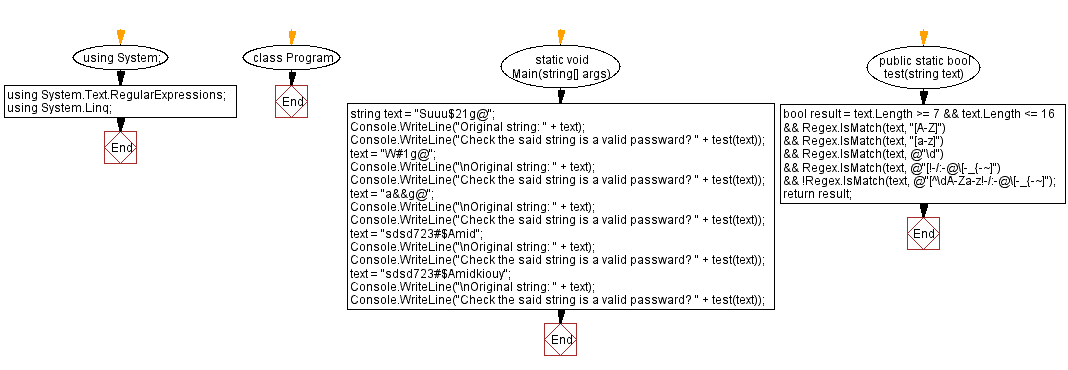
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Possible Palindromes from string of characters.
Next C# Sharp Exercise: Check two strings contain the same character pattern.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.