C#: Check a number is prime number or not
Write a program in C# Sharp to check whether a number is prime or not using recursion.
Visual Presentation:
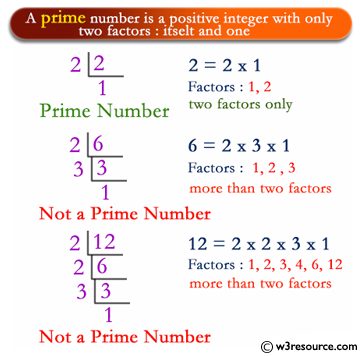
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'RecExercise7'
class RecExercise7
{
// Main method
public static int Main()
{
int n1, primeNo;
// Prompt user to input a positive number
Console.WriteLine("\n\n Recursion : Check a number is prime number or not :");
Console.WriteLine("--------------------------------------------------------");
Console.Write(" Input any positive number : ");
n1 = Convert.ToInt32(Console.ReadLine());
// Call the function 'checkForPrime' to determine if the input number is prime
primeNo = checkForPrime(n1, n1 / 2);
// Output whether the number is prime or not
if (primeNo == 1)
Console.Write(" The number {0} is a prime number. \n\n", n1);
else
Console.WriteLine(" The number {0} is not a prime number. \n\n", n1);
return 0;
}
// Recursive function to check for prime number
static int checkForPrime(int n1, int i)
{
if (i == 1)
{
return 1; // Base case: If i reaches 1, it is a prime number
}
else
{
// If the number is divisible by i, it's not a prime number
if (n1 % i == 0)
return 0;
else
return checkForPrime(n1, i - 1); // Recursive call: Call checkForPrime itself with decreased i
}
}
}
Sample Output:
Recursion : Check a number is prime number or not : -------------------------------------------------------- Input any positive number : 5 The number 5 is a prime number.
Flowchart :
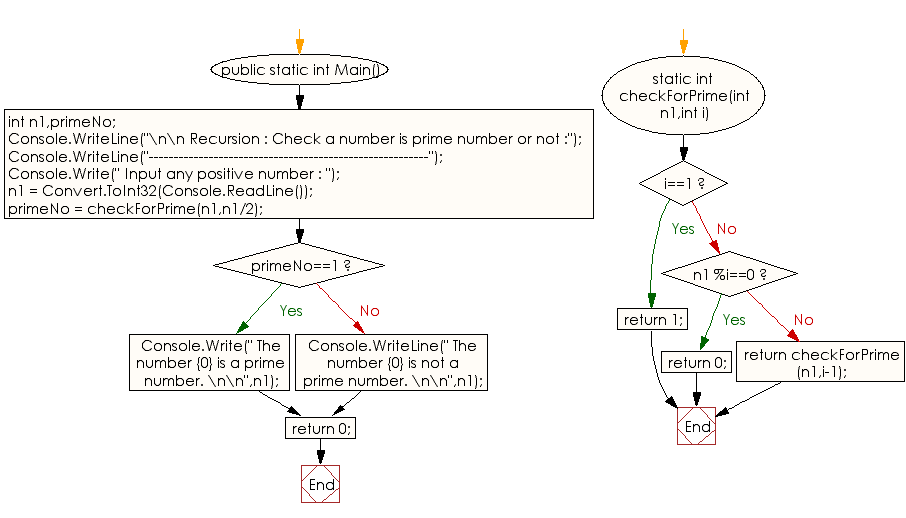
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to print even or odd numbers in a given range using recursion.
Next: Write a program in C# Sharp to Check whether a given String is Palindrome or not using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.