C#: Merge sort
Write a C# Sharp program to sort a list of elements using Merge sort.
According to Wikipedia "Merge sort (also commonly spelled mergesort) is an O (n log n) comparison-based sorting algorithm. Most implementations produce a stable sort, which means that the implementation preserves the input order of equal elements in the sorted output."
Algorithm :Conceptually, a merge sort works as follows :
- Divide the unsorted list into n sublists, each containing 1 element (a list of 1 element is considered sorted).
- Repeatedly merge sublists to produce new sorted sublists until there is only 1 sublist remaining. This will be the sorted list.
An example of merge sort :
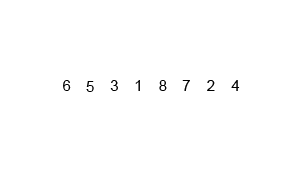
Merge Sort: Pictorial Presentation
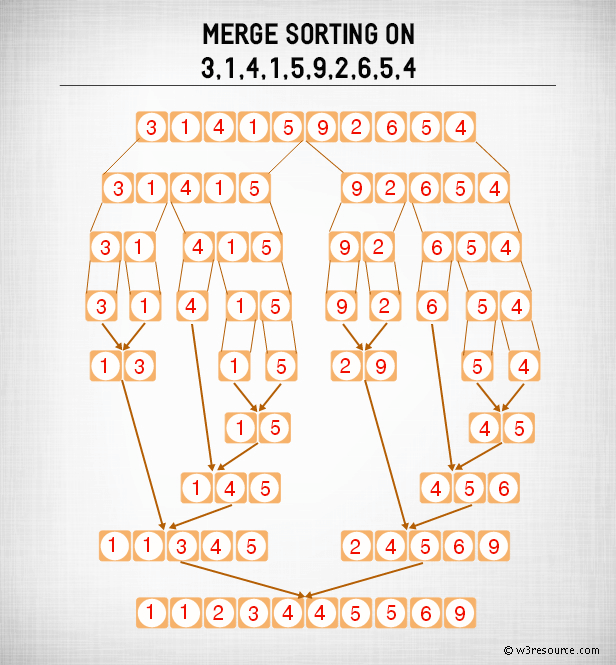
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Merge_sort
{
class Program
{
static void Main(string[] args)
{
// Initialize lists for unsorted and sorted elements
List<int> unsorted = new List<int>();
List<int> sorted;
// Create a random number generator
Random random = new Random();
Console.WriteLine("Original array elements:");
// Generate and display random unsorted elements
for(int i = 0; i < 10; i++)
{
unsorted.Add(random.Next(0, 100)); // Add a random number to the unsorted list
Console.Write(unsorted[i] + " "); // Display the added number
}
Console.WriteLine();
// Perform Merge Sort on the unsorted list
sorted = MergeSort(unsorted);
// Display the sorted array elements
Console.WriteLine("Sorted array elements:");
foreach (int x in sorted)
{
Console.Write(x + " ");
}
Console.Write("\n");
}
// Method to perform Merge Sort
private static List<int> MergeSort(List<int> unsorted)
{
if (unsorted.Count <= 1)
return unsorted; // Base case: return the list if it has only one element
// Divide the unsorted list into two halves
List<int> left = new List<int>();
List<int> right = new List<int>();
int middle = unsorted.Count / 2;
for (int i = 0; i < middle; i++) // Split the unsorted list into left
{
left.Add(unsorted[i]);
}
for (int i = middle; i < unsorted.Count; i++) // Split the unsorted list into right
{
right.Add(unsorted[i]);
}
// Recursively perform Merge Sort on the divided lists
left = MergeSort(left);
right = MergeSort(right);
// Merge the sorted halves
return Merge(left, right);
}
// Method to merge two sorted lists
private static List<int> Merge(List<int> left, List<int> right)
{
List<int> result = new List<int>();
// Compare elements from both lists and merge them in sorted order
while (left.Count > 0 || right.Count > 0)
{
if (left.Count > 0 && right.Count > 0)
{
if (left.First() <= right.First()) // Compare the first elements of both lists
{
result.Add(left.First());
left.Remove(left.First()); // Remove the added element from the list
}
else
{
result.Add(right.First());
right.Remove(right.First()); // Remove the added element from the list
}
}
else if (left.Count > 0)
{
result.Add(left.First());
left.Remove(left.First());
}
else if (right.Count > 0)
{
result.Add(right.First());
right.Remove(right.First());
}
}
return result; // Return the merged and sorted list
}
}
}
Sample Output:
Original array elements: 79 69 9 95 65 49 65 40 27 95 Sorted array elements: 9 27 40 49 65 65 69 79 95 95
Flowchart:
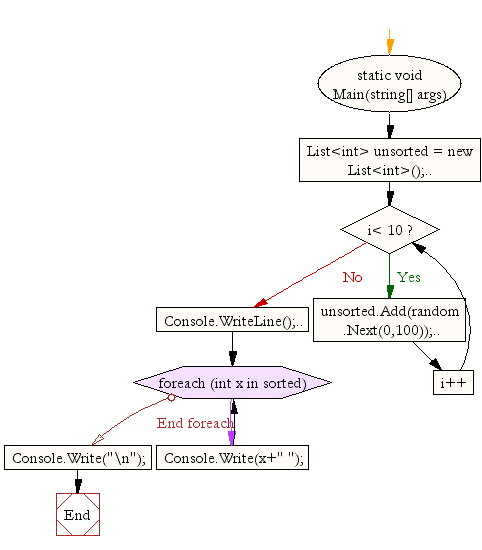
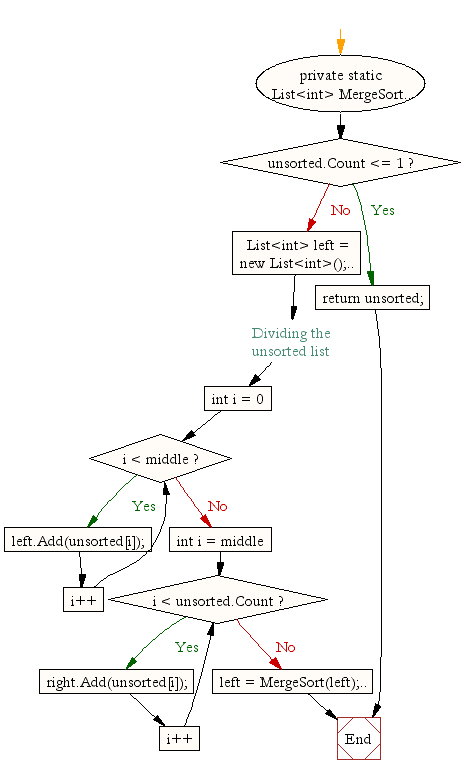
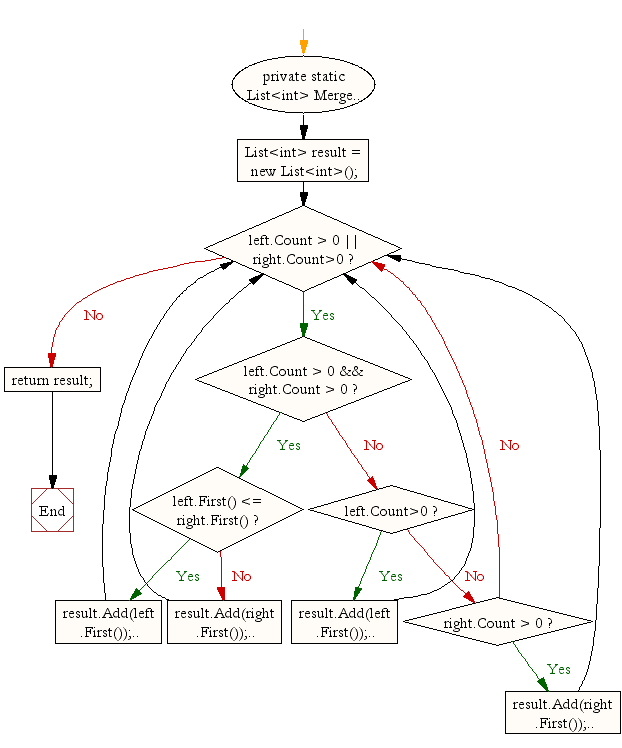
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to sort a list of elements using Insertion sort.
Next: Write a C# Sharp program to sort a list of elements using Permutation sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.