C#: Top and bottom elements of a stack
Write a C# program to find the top and bottom elements of a given stack.
Sample Solution:
C# Code:
using System;
using System.Collections.Generic;
// Implementation of a Stack data structure
public class Stack
{
private int[] items; // Array to hold stack elements
private int top; // Index representing the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Initializing the array with the given size
top = -1; // Initializing top to -1, indicating an empty stack
}
// Method to check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Returns true if top is -1 (empty stack), otherwise false
}
// Method to check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Returns true if top is at the last index of the array (full stack)
}
// Method to push an element onto the stack
public void Push(int item)
{
if (IsFull())
{
Console.WriteLine("Stack Full!"); // Displays a message if the stack is full
return;
}
items[++top] = item; // Inserts the item at the incremented top index
}
// Method to pop an element from the stack
public int Pop()
{
if (IsEmpty())
{
Console.WriteLine("Stack underflow"); // Displays a message if the stack is empty
return -1;
}
return items[top--]; // Removes and returns the top element by decrementing top
}
// Method to peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty())
{
Console.WriteLine("Stack is empty"); // Displays a message if the stack is empty
return -1;
}
return items[top]; // Returns the element at the top index without removing it
}
// Method to get the top element of the stack
public static int GetTopElement(Stack stack)
{
if (stack == null || stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return -1;
}
return stack.Peek();
}
// Method to get the bottom element of the stack
public static int GetBottomElement(Stack stack)
{
if (stack == null || stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return -1;
}
Stack tempStack = new Stack(Size(stack));
while (!stack.IsEmpty())
{
tempStack.Push(stack.Pop());
}
int bottomElement = tempStack.Peek();
while (!tempStack.IsEmpty())
{
stack.Push(tempStack.Pop());
}
return bottomElement;
}
// Method to get the current size of the stack
public static int Size(Stack stack)
{
return stack.top + 1; // Returns the number of elements in the stack
}
// Method to display the stack elements
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " "); // Displays each element in the stack
}
}
}
// Main class to demonstrate the functionality of the Stack class
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize a stack:");
Stack stack = new Stack(10); // Creating a stack with a size of 10
Console.WriteLine("Input some elements onto the stack:");
stack.Push(10);
stack.Push(50);
stack.Push(30);
stack.Push(40);
stack.Push(50);
stack.Push(50);
Stack.Display(stack); // Displaying the elements in the original stack
// Retrieving the top and bottom elements of the stack
int topElement = Stack.GetTopElement(stack);
int bottomElement = Stack.GetBottomElement(stack);
// Displaying the top and bottom elements of the stack
Console.WriteLine("\n\nTop element of the said stack is {0}", topElement);
Console.WriteLine("Bottom element of the said stack is {0}", bottomElement);
}
}
Sample Output:
Initialize a stack: Input some elements onto the stack: Stack elements: 50 50 40 30 50 10 Top element of the said stack is 50 Bottom element of the said stack is 10
Flowchart:
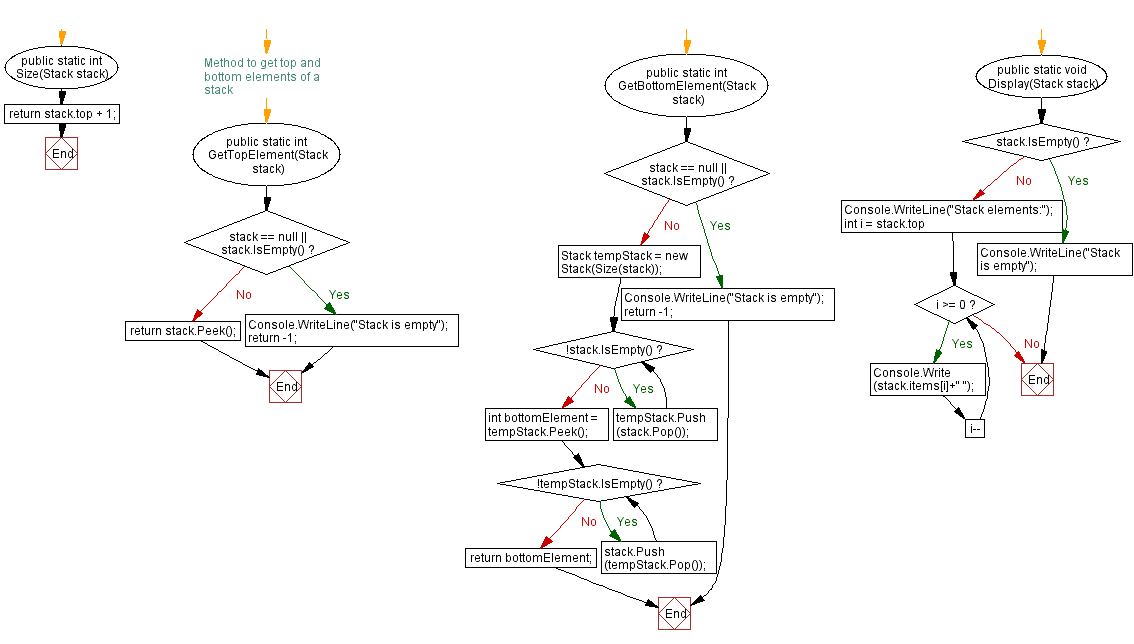
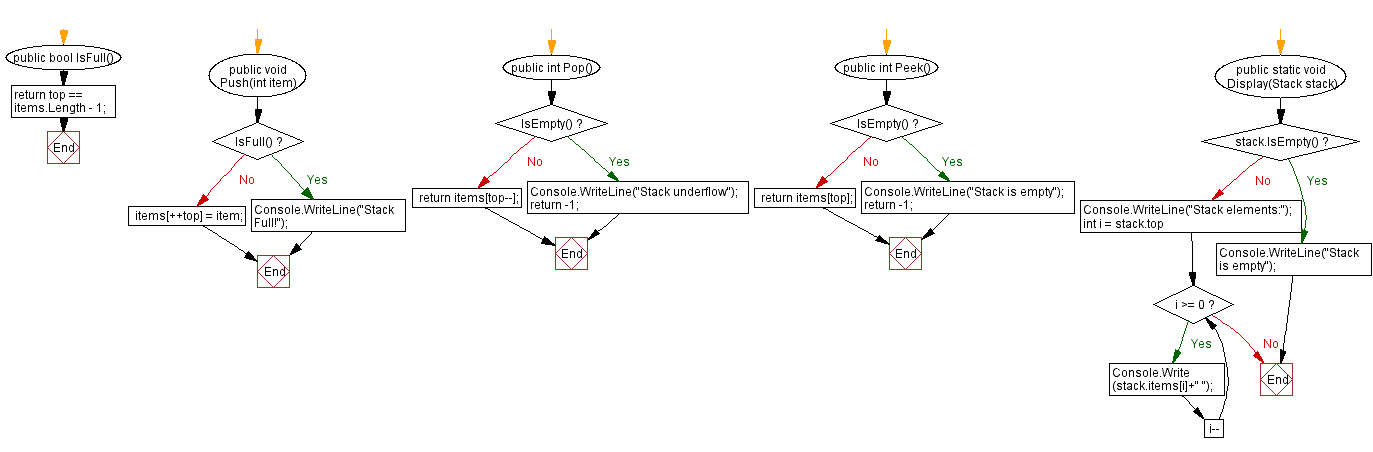
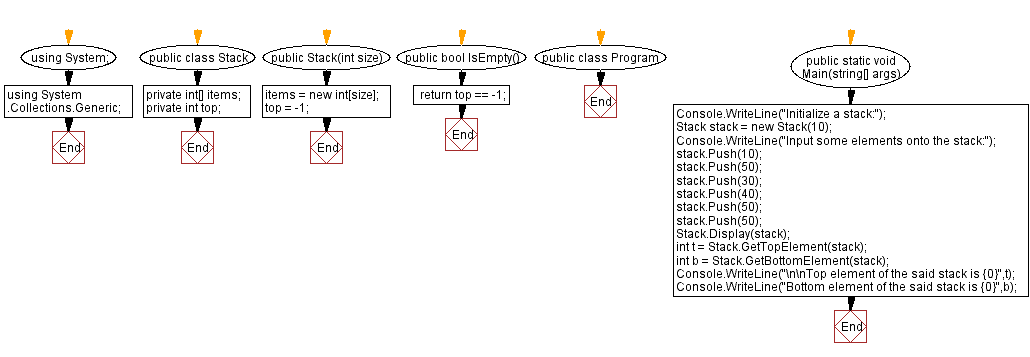
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Remove duplicates from a given stack.
Next: Rotate the stack elements to the left.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.