C#: Replace lowercase characters by uppercase and vice-versa
Write a C# Sharp program to read a sentence and replace lowercase characters with uppercase and vice-versa.
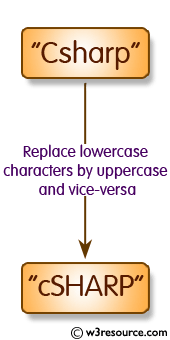
Sample Solution:-
C# Sharp Code:
using System;
// Define the exercise15 class
public class exercise15
{
// Main method - entry point of the program
public static void Main()
{
string str1; // Declare a string variable to store input
char[] arr1; // Declare a character array variable
int l, i; // Declare integer variables
l = 0; // Initialize variable 'l' to 0
char ch; // Declare a character variable 'ch'
// Prompt the user for input
Console.Write("\n\nReplace lowercase characters by uppercase and vice-versa :\n");
Console.Write("--------------------------------------------------------------\n");
Console.Write("Input the string : ");
str1 = Console.ReadLine(); // Read the input string from the user
l = str1.Length; // Get the length of the input string
arr1 = str1.ToCharArray(0, l); // Convert the string into a character array
Console.Write("\nAfter conversion, the string is : ");
// Loop through each character in the array
for (i = 0; i < l; i++)
{
ch = arr1[i]; // Get the current character
// Check whether the character is lowercase
if (Char.IsLower(ch))
{
Console.Write(Char.ToUpper(ch)); // Convert lowercase character to uppercase and print
}
else
{
Console.Write(Char.ToLower(ch)); // Convert uppercase character to lowercase and print
}
}
Console.Write("\n\n");
}
}
Sample Output:
Replace lowercase characters by uppercase and vice-versa : -------------------------------------------------------------- Input the string : w3RESOURCE After conversion, the string is : W3resource
Flowchart:
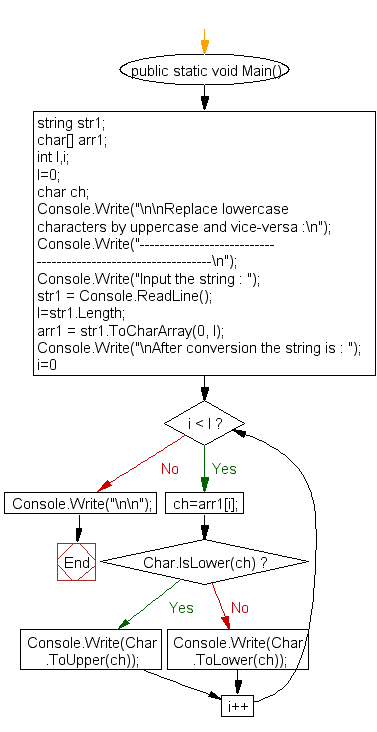
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a given substring is present in the given string
Next: Write a program in C# Sharp to check the username and password.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.