C#: Search the position of a substring within a string
Write a program in C# Sharp to search for the position of a substring within a string.
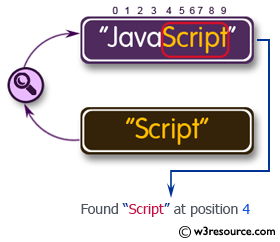
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise17 class
public class Exercise17
{
// Main method - entry point of the program
public static void Main()
{
string str1; // Declare a variable to store the main string
string findstr; // Declare a variable to store the substring to be found
// Prompt the user to search for a substring within a string
Console.Write("\n\nSearch the position of a substring within a string :\n");
Console.Write("-------------------------------------------------------\n");
// Ask the user to input a string
Console.Write("Input a String: ");
str1 = Console.ReadLine();
// Ask the user to input a substring to be found in the string
Console.Write("Input a substring to be found in the string: ");
findstr = Console.ReadLine();
// Find the index of the substring in the main string
int index = str1.IndexOf(findstr);
// Check if the substring is found or not
if (index < 0)
{
Console.WriteLine("The substring was not found in the given string.\n");
}
else
{
// Display the position/index where the substring was found in the main string
Console.WriteLine("Found '{0}' in '{1}' at position {2}",
findstr, str1, index);
}
}
}
Sample Output:
Search the position of a substing within a string : ------------------------------------------------------- Input a String: Welcome to w3resource.com Input a substring to be found in the string: w3resource Found 'w3resource' in 'Welcome to w3resource.com' at position 11
Flowchart:
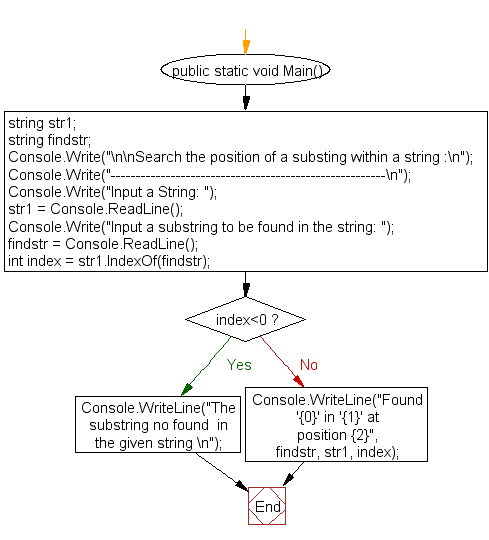
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to check the username and password.
Next: Write a program in C# Sharp to check whether a character is an alphabet and not and if so, go to check for the case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.