C#: Reverse an integer and add with the original number
Write a C# Sharp program to reverse a positive integer and add the reversed number to the original number.
Sample Data:
(456) - > 456654
(-123) -> "Input a positive number."
(10000) -> 1000000001
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize an integer variable
int n = 567;
// Display the original number
Console.WriteLine("Original number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
// Change the value of n to a negative number
n = -123;
// Display the new original number
Console.WriteLine("\nOriginal number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
// Change the value of n to a large number
n = 10000;
// Display the new original number
Console.WriteLine("\nOriginal number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
}
// Define a method 'test' that takes an integer argument 'n' and returns a string
public static string test(int n)
{
// Check if the number is greater than zero
if (n > 0)
{
// Convert the integer to a string, reverse it, convert back to string, and concatenate with the original number
return n + new string(n.ToString().Reverse().ToArray());
}
// Return a message if the input is not a positive number
return "Input a positive number.";
}
}
}
Sample Output:
Original number: 567 Reverse the said integer and add with the original number- 567765 Original number: -123 Reverse the said integer and add with the original number- Input a positive number. Original number: 10000 Reverse the said integer and add with the original number- 1000000001
Flowchart :
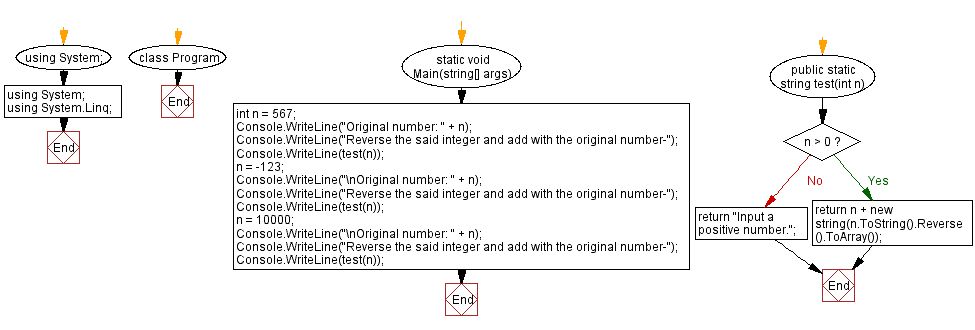
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize an integer variable
int n = 567;
// Display the original number
Console.WriteLine("Original number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
// Change the value of n to a negative number
n = -123;
// Display the new original number
Console.WriteLine("\nOriginal number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
// Change the value of n to a large number
n = 10000;
// Display the new original number
Console.WriteLine("\nOriginal number: " + n);
// Display the action about to be performed
Console.WriteLine("Reverse the said integer and add with the original number-");
// Invoke the 'test' method and display the result
Console.WriteLine(test(n));
}
// Define a method 'test' that takes an integer argument 'n' and returns a string
public static string test(int n)
{
// Check if the number is greater than zero
if (n > 0)
{
// Convert the integer to a string and concatenate it with its reverse
return n.ToString() + string.Concat(n.ToString().Reverse());
}
// Return a message if the input is not a positive number
return "Input a positive number.";
}
}
}
Sample Output:
Original number: 567 Reverse the said integer and add with the original number- 567765 Original number: -123 Reverse the said integer and add with the original number- Input a positive number. Original number: 10000 Reverse the said integer and add with the original number- 1000000001
Flowchart :
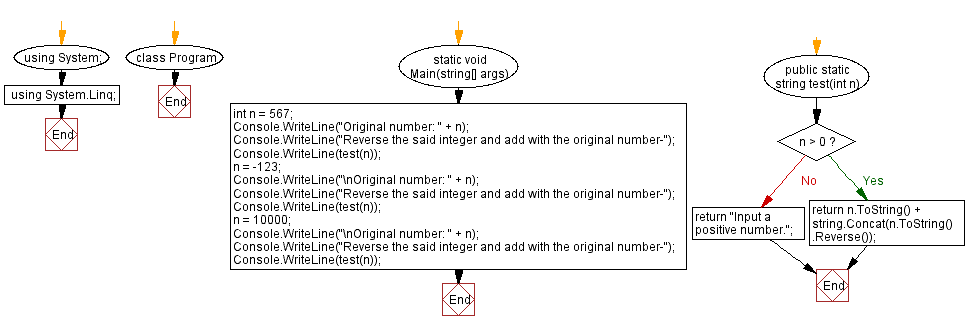
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Check if a string is an anagram of another given string.
Next: Count the number of duplicate characters in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.