C#: Alphabet position in a string
C# Sharp String: Exercise-62 with Solution
Write a C# Sharp program that displays all the characters with their respective numbers from a string.
Sample Data:
("C Sharp") -> " 3 19 8 1 18 16"
("The quick brown fox jumps over the lazy dog.") -> " 20 8 5 17 21 9 3 11 2 18 15 23 14 6 15 24
10 21 13 16 19 15 22 5 18 20 8 5 12 1 26 25 4 15 7"
("HTML Tutorial") -> " 8 20 13 12 20 21 20 15 18 9 1 12 "
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "The quick brown fox jumps over the lazy dog.";
// Display the original string
Console.WriteLine("Original string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
// Change the value of text
text = "C Sharp";
// Display the new original string
Console.WriteLine("\nOriginal string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
// Change the value of text
text = "HTML Tutorial";
// Display the new original string
Console.WriteLine("\nOriginal string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns a string
public static string test(string text)
{
// Convert the characters to lowercase, filter only alphabetic characters, calculate their alphabet positions,
// and convert these positions to an array of integers
var char_positions = text.ToLower().Where(ch => Char.IsLetter(ch)).
Select(ch => (int)ch % 32).ToArray();
// Join the array elements into a string separated by spaces and return it
return string.Join(" ", char_positions);
}
}
}
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Alphabet position in the said string: 20 8 5 17 21 9 3 11 2 18 15 23 14 6 15 24 10 21 13 16 19 15 22 5 18 20 8 5 12 1 26 25 4 15 7 Original string: C Sharp Alphabet position in the said string: 3 19 8 1 18 16 Original string: HTML Tutorial Alphabet position in the said string: 8 20 13 12 20 21 20 15 18 9 1 12
Flowchart :
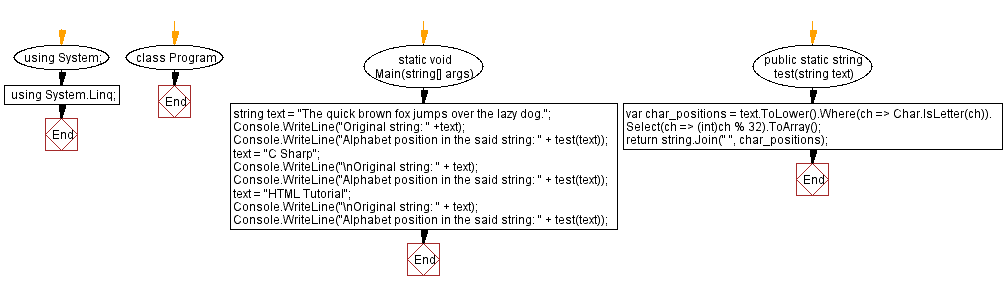
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "The quick brown fox jumps over the lazy dog.";
// Display the original string
Console.WriteLine("Original string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
// Change the value of text
text = "C Sharp";
// Display the new original string
Console.WriteLine("\nOriginal string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
// Change the value of text
text = "HTML Tutorial";
// Display the new original string
Console.WriteLine("\nOriginal string: " + text);
// Display the alphabet positions of characters in the string
Console.WriteLine("Alphabet position in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns a string
public static string test(string text)
{
// Use LINQ to filter only alphabetic characters, calculate their alphabet positions (A=1, B=2,...,Z=26),
// and convert these positions to a string separated by spaces
return String.Join(" ", text.Where(ch => char.IsLetter(ch)).Select(ch => (int)ch % 32));
}
}
}
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Alphabet position in the said string: 20 8 5 17 21 9 3 11 2 18 15 23 14 6 15 24 10 21 13 16 19 15 22 5 18 20 8 5 12 1 26 25 4 15 7 Original string: C Sharp Alphabet position in the said string: 3 19 8 1 18 16 Original string: HTML Tutorial Alphabet position in the said string: 8 20 13 12 20 21 20 15 18 9 1 12
Flowchart :
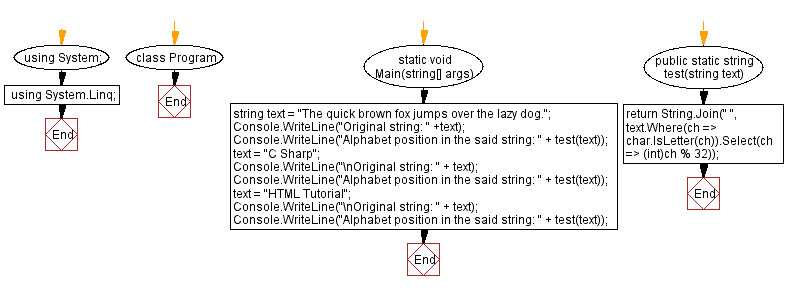
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Count Occurrences of a substring in a string.
Next C# Sharp Exercise: Number of times a substring appeared in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics