C#: Count a total number of vowel or consonant
Write a C# Sharp program to count the number of vowels or consonants in a string.
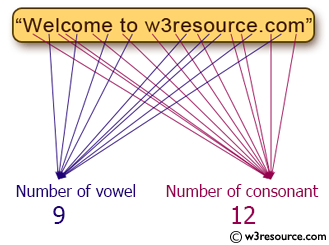
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise9 class
public class Exercise9
{
// Main method - entry point of the program
public static void Main()
{
string str; // Declare a string variable to hold user input
int i, len, vowel, cons; // Declare variables for iteration, string length, vowel count, and consonant count
// Prompt the user to input a string
Console.Write("\n\nCount total number of vowel or consonant:\n");
Console.Write("----------------------------------------------\n");
Console.Write("Input the string: ");
str = Console.ReadLine(); // Read the input string from the user
vowel = 0; // Initialize vowel count to zero
cons = 0; // Initialize consonant count to zero
len = str.Length; // Get the length of the input string
// Loop through each character of the string to count vowels and consonants
for (i = 0; i < len; i++)
{
// Check if the character is a vowel (both lowercase and uppercase)
if (str[i] == 'a' || str[i] == 'e' || str[i] == 'i' || str[i] == 'o' || str[i] == 'u' ||
str[i] == 'A' || str[i] == 'E' || str[i] == 'I' || str[i] == 'O' || str[i] == 'U')
{
vowel++; // Increment the vowel count
}
// Check if the character is an alphabet (both lowercase and uppercase) but not a vowel
else if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z'))
{
cons++; // Increment the consonant count
}
}
// Display the total number of vowels and consonants in the input string
Console.Write("\nThe total number of vowels in the string is: {0}\n", vowel);
Console.Write("The total number of consonants in the string is: {0}\n\n", cons);
}
}
Sample Output:
Count total number of vowel or consonant : ---------------------------------------------- Input the string : Welcome to w3resource.com The total number of vowel in the string is : 9 The total number of consonant in the string is : 12
Flowchart:
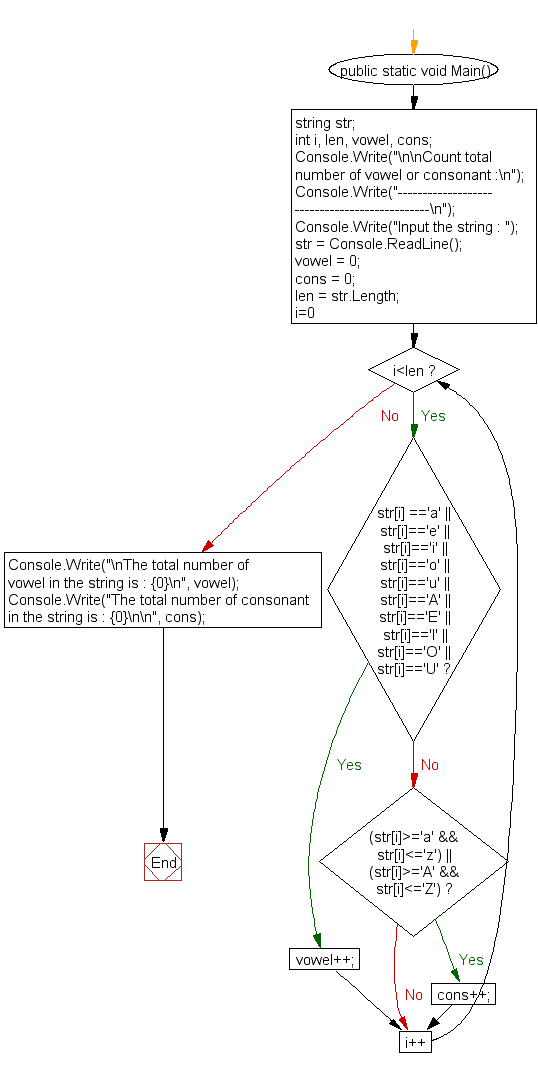
C# Sharp Practice online:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to copy one string to another string.
Next: Write a program in C# Sharp to find maximum occurring character in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.